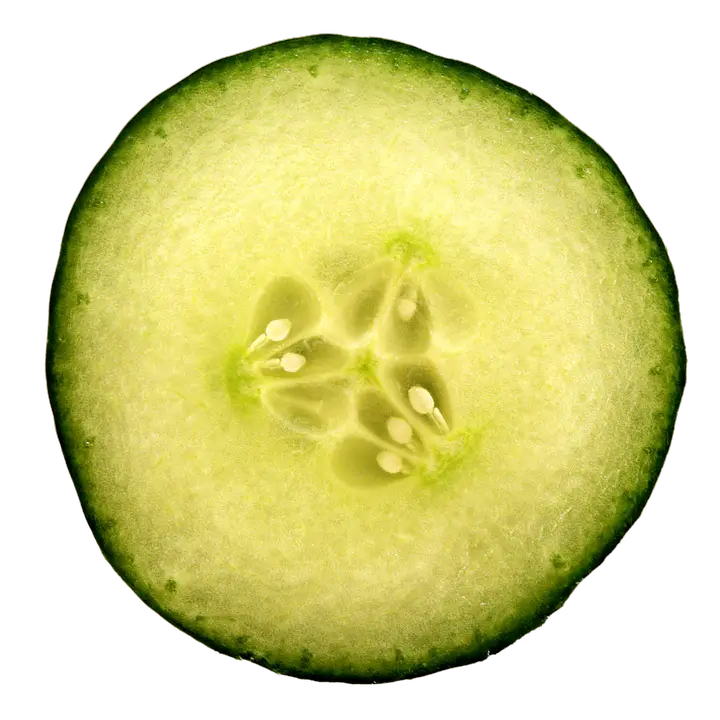
Text parsing is a common task in programming that involves breaking down a piece of text into its component parts. This can be useful for extracting specific information from a larger body of text, such as parsing a CSV file or extracting data from a website.
One powerful tool for text parsing in Python is the split() method. This method allows you to split a string into a list of substrings based on a delimiter. By mastering the split() method, you can efficiently parse text and extract the information you need.
The split() method takes a delimiter as an argument, which is used to determine where to split the string. For example, if you have a string “apple,banana,orange” and you use a comma as the delimiter, the split() method will return a list with three elements: [“apple”, “banana”, “orange”].
Here’s an example of how you can use the split() method to parse a CSV file:
“` python
csv_string = “name,age,city\nAlice,25,New York\nBob,30,San Francisco”
lines = csv_string.split(“\n”)
for line in lines:
values = line.split(“,”)
print(values)
“`
In this example, we first split the CSV string into lines using the newline character “\n”. Then, we iterate over each line and split it into values using the comma as the delimiter. Finally, we print out the values for each line.
The split() method is flexible and allows you to also specify a maximum number of splits to perform. For example, if you only want to split a string into two parts, you can pass a second argument to the split() method:
“` python
sentence = “The quick brown fox jumps over the lazy dog”
words = sentence.split(” “, 2)
print(words)
“`
In this example, we split the sentence into a maximum of two parts using the space character as the delimiter. The output will be [“The”, “quick”, “brown fox jumps over the lazy dog”].
While the split() method is powerful, it’s important to be mindful of the delimiter you choose. If the delimiter appears within the text you are parsing, it can lead to incorrect results. In such cases, you may need to use more advanced parsing techniques or regular expressions.
In conclusion, mastering the split() method in Python can greatly enhance your text parsing capabilities. By understanding how to use this method effectively, you can efficiently extract information from text data and streamline your programming tasks. Practice using the split() method with different delimiters and scenarios to become proficient in text parsing with Python.