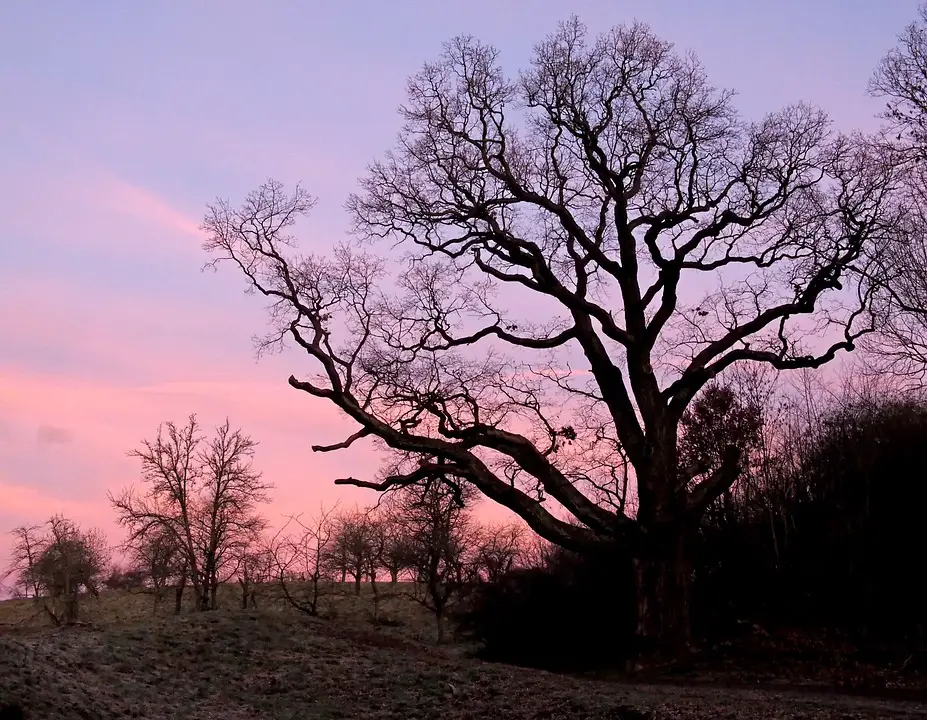
Python’s split() function is a powerful tool that allows you to easily extract data from strings. Whether you are working with text files, web data, or any other type of string data, the split() function can help you quickly and efficiently parse and extract the information you need. In this article, we will explore how to use the split() function in Python to extract data from strings.
The split() function in Python is used to split a string into a list of substrings based on a specified delimiter. By default, the split() function splits the string using whitespace as the delimiter, but you can also specify a custom delimiter as an argument to the function.
Here is the basic syntax of the split() function in Python:
“` python
string.split(separator)
“`
In this syntax, “string” is the string that you want to split, and “separator” is the delimiter that you want to use to split the string. When you call the split() function on a string, it will return a list of substrings that were separated by the specified delimiter.
Let’s look at an example to see how the split() function works in practice. Suppose we have a string that contains a list of names separated by commas, like this:
“` python
names = “Alice,Bob,Charlie,David”
“`
If we want to extract each name from this string and store them in a list, we can use the split() function with a comma as the delimiter:
“` python
name_list = names.split(“,”)
print(name_list)
“`
When we run this code, the output will be:
“` python
[‘Alice’, ‘Bob’, ‘Charlie’, ‘David’]
“`
As you can see, the split() function has split the original string into a list of substrings, with each name being stored as a separate element in the list.
In addition to specifying a single delimiter, you can also use the split() function with multiple delimiters to extract data from more complex strings. For example, if you have a string that contains both commas and spaces as delimiters, you can pass a list of delimiters to the split() function:
“` python
data = “Alice, Bob Charlie David”
data_list = data.split([” “, “,”])
print(data_list)
“`
In this example, the split() function will split the string based on both spaces and commas, resulting in the following output:
“` python
[‘Alice’, ‘Bob’, ‘Charlie’, ‘David’]
“`
By using the split() function in Python, you can easily extract data from strings and manipulate it in a variety of ways. Whether you are processing text files, parsing web data, or performing any other string manipulation task, the split() function is a valuable tool that can help you efficiently extract the information you need.