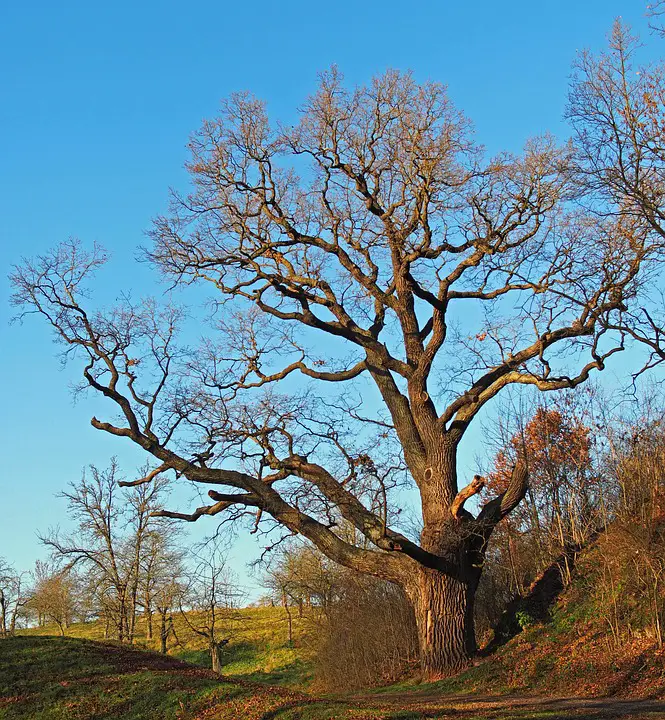
Python’s range function is a powerful tool that allows you to easily generate a sequence of numbers for looping and iteration. By using range, you can efficiently create loops that iterate over a specified range of numbers without having to manually create a list of numbers.
To use the range function, you simply need to provide it with the start and stop values of the range you want to generate. The function will then return a sequence of numbers starting from the start value up to, but not including, the stop value. For example, if you call range(1, 5), you will get the sequence [1, 2, 3, 4].
One of the key benefits of using range for looping and iteration is that it is memory efficient. Instead of creating a list of numbers in memory, range generates the numbers on the fly as you iterate over them. This means that you can easily work with large ranges without worrying about running out of memory.
Another advantage of using range is that it is a more Pythonic way of creating loops. Instead of using a while loop or manually creating a list of numbers, you can simply use the range function to generate the sequence of numbers you need. This makes your code cleaner and more readable.
Here are a few examples of how you can use range for efficient looping and iteration in Python:
1. Using range in a for loop:
“`
for i in range(5):
print(i)
“`
This will iterate over the numbers 0, 1, 2, 3, and 4.
2. Using range with a start and stop value:
“`
for i in range(1, 5):
print(i)
“`
This will iterate over the numbers 1, 2, 3, and 4.
3. Using range with a step value:
“`
for i in range(1, 10, 2):
print(i)
“`
This will iterate over the numbers 1, 3, 5, 7, and 9, with a step size of 2.
Overall, Python’s range function is a powerful tool for efficiently creating loops and iterations over a sequence of numbers. By using range, you can write cleaner and more readable code while also conserving memory when working with large ranges. So next time you need to iterate over a range of numbers, consider using Python’s range function for a more efficient solution.