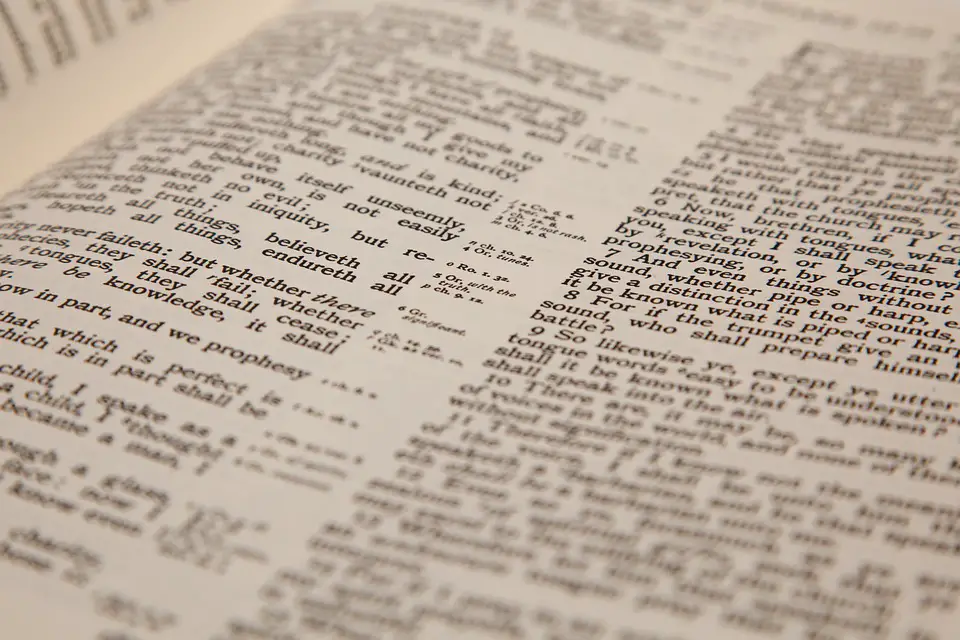
JavaScript filter is a powerful method that allows you to streamline your code by efficiently manipulating arrays. By using filter, you can easily create new arrays that only contain elements that meet certain criteria, without having to write complex loops or conditional statements.
Here are some tips on how to use JavaScript filter effectively:
1. Understand the basics of filter: The filter method creates a new array with all elements that pass a certain test implemented by the provided function. The syntax for using filter is as follows:
“`javascript
const newArray = array.filter(callback(element, index, array));
“`
2. Write a callback function: The callback function is a crucial part of the filter method. It takes in three arguments: the current element being processed, its index in the array, and the array itself. The function should return true if the element should be included in the new array, and false if it should be excluded.
“`javascript
const numbers = [1, 2, 3, 4, 5];
const evenNumbers = numbers.filter(number => number % 2 === 0);
console.log(evenNumbers); // Output: [2, 4]
“`
3. Use filter to remove unwanted elements: One common use case for filter is to remove elements from an array that do not meet certain criteria. For example, you can use filter to remove all negative numbers from an array:
“`javascript
const numbers = [-3, 5, -1, 8, -2];
const positiveNumbers = numbers.filter(number => number >= 0);
console.log(positiveNumbers); // Output: [5, 8]
“`
4. Combine filter with other array methods: Filter can be combined with other array methods like map, reduce, and forEach to perform more complex operations on arrays. For example, you can use filter to first select certain elements from an array, and then use map to transform those elements:
“`javascript
const words = [‘hello’, ‘world’, ‘goodbye’];
const filteredWords = words.filter(word => word.length > 5).map(word => word.toUpperCase());
console.log(filteredWords); // Output: [“GOODBYE”]
“`
5. Use filter with objects: Filter can also be used with arrays of objects. You can pass in a callback function that checks for certain properties or values in the objects and creates a new array with only the objects that meet the criteria:
“`javascript
const users = [
{ name: ‘Alice’, age: 26 },
{ name: ‘Bob’, age: 30 },
{ name: ‘Charlie’, age: 22 }
];
const adults = users.filter(user => user.age >= 18);
console.log(adults); // Output: [{ name: ‘Alice’, age: 26 }, { name: ‘Bob’, age: 30 }]
“`
In conclusion, JavaScript filter is a versatile method that can help you streamline your code by efficiently manipulating arrays. By understanding how to use filter effectively and combining it with other array methods, you can write cleaner and more concise code that is easier to maintain and understand. Experiment with filter in your own projects to see how it can improve the efficiency and readability of your code.