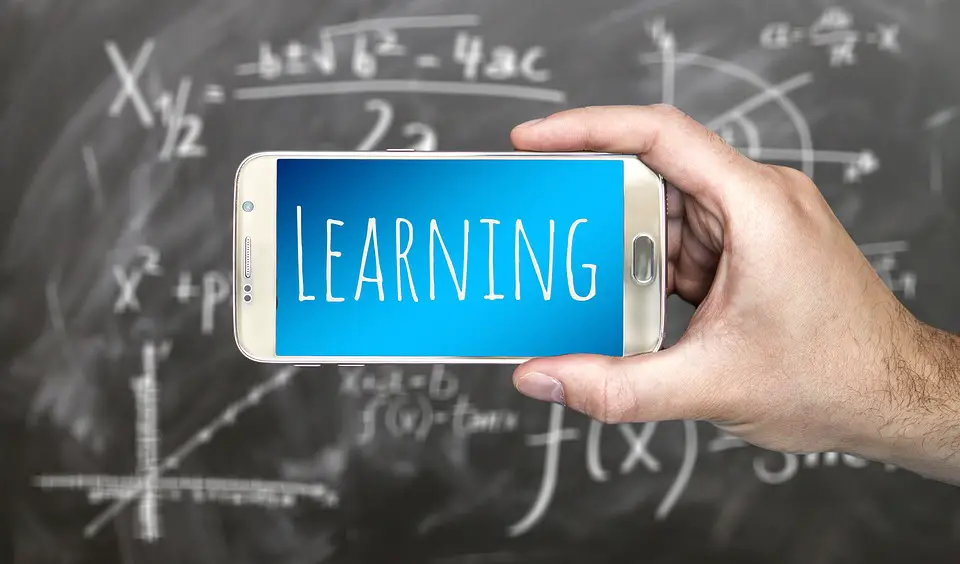
Python is a versatile and powerful programming language that is widely used in various fields such as web development, data analysis, and automation. One of the key features that makes Python so popular is its built-in function called `range`.
The `range` function in Python is used to generate a sequence of numbers within a specified range. It is commonly used in for loops to iterate over a sequence of numbers. The syntax of the `range` function is as follows:
“`
range(start, stop, step)
“`
Where `start` is the starting value of the sequence, `stop` is the ending value of the sequence (not inclusive), and `step` is the increment between each number in the sequence.
Harnessing the power of the `range` function in Python can greatly improve the efficiency and readability of your code. Here are some ways in which you can leverage the `range` function for improved programming:
1. Generating a sequence of numbers:
The most common use of the `range` function is to generate a sequence of numbers within a specified range. For example, you can use `range(1, 10)` to generate a sequence of numbers from 1 to 9.
2. Iterating over a sequence:
You can use the `range` function in a for loop to iterate over a sequence of numbers. For example, the following code snippet prints all the numbers from 1 to 9:
“`
for i in range(1, 10):
print(i)
“`
3. Specifying a step value:
You can also specify a step value in the `range` function to generate a sequence with a specific increment between each number. For example, `range(1, 10, 2)` will generate a sequence of numbers with an increment of 2 between each number.
4. Generating a reverse sequence:
You can use a negative step value in the `range` function to generate a reverse sequence of numbers. For example, `range(10, 1, -1)` will generate a sequence of numbers from 10 to 2 in reverse order.
5. Creating a list of numbers:
You can convert the output of the `range` function into a list using the `list` function. For example, `list(range(1, 10))` will create a list of numbers from 1 to 9.
In conclusion, the `range` function in Python is a powerful tool that can greatly enhance the functionality and efficiency of your code. By harnessing the power of the `range` function, you can easily generate sequences of numbers, iterate over them, specify custom increments, generate reverse sequences, and create lists of numbers. Next time you are working on a Python project, don’t forget to leverage the `range` function for improved programming.