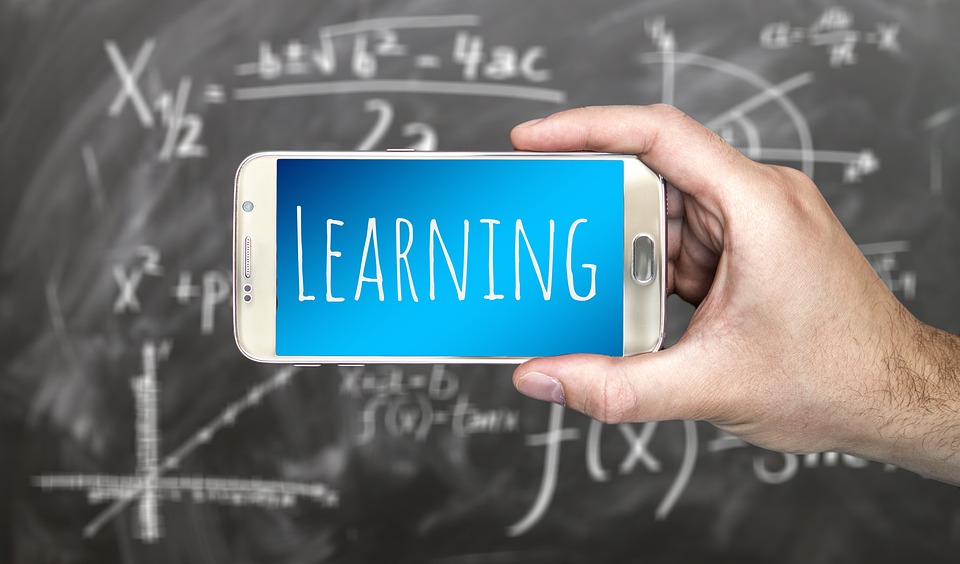
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing-state and mutable data. One of the key concepts in functional programming is the use of higher-order functions, which are functions that can take other functions as arguments or return them as results. Python, a widely-used programming language, supports functional programming concepts through its built-in functions such as map, filter, and reduce.
In this article, we will focus on Python’s map function and how it can be used to harness the power of functional programming. The map function is a higher-order function that applies a given function to each item in an iterable (such as a list, tuple, or set) and returns a new iterable with the results. This allows for a concise and expressive way to transform data without the need for loops or mutable variables.
One of the key benefits of using the map function is that it promotes a more declarative and readable coding style. By separating the logic of transforming data into a separate function, the code becomes more modular and easier to understand. This can lead to fewer bugs and easier maintenance of the codebase.
Let’s take a look at an example to illustrate the power of the map function in Python:
“` python
# Define a function to square a number
def square(x):
return x * x
# Create a list of numbers
numbers = [1, 2, 3, 4, 5]
# Use the map function to square each number in the list
squared_numbers = map(square, numbers)
# Convert the map object to a list
squared_numbers_list = list(squared_numbers)
print(squared_numbers_list)
“`
In this example, we define a function called square that takes a number as input and returns the square of that number. We then create a list of numbers and use the map function to apply the square function to each number in the list. Finally, we convert the map object to a list and print the result.
By using the map function, we were able to transform the data in a concise and readable way without the need for explicit loops or mutable variables. This can lead to more maintainable and bug-free code.
In conclusion, Python’s map function is a powerful tool for harnessing the power of functional programming in your code. By using higher-order functions like map, you can write more declarative and expressive code that is easier to understand and maintain. So next time you need to transform data in your Python code, consider using the map function to take advantage of the benefits of functional programming.