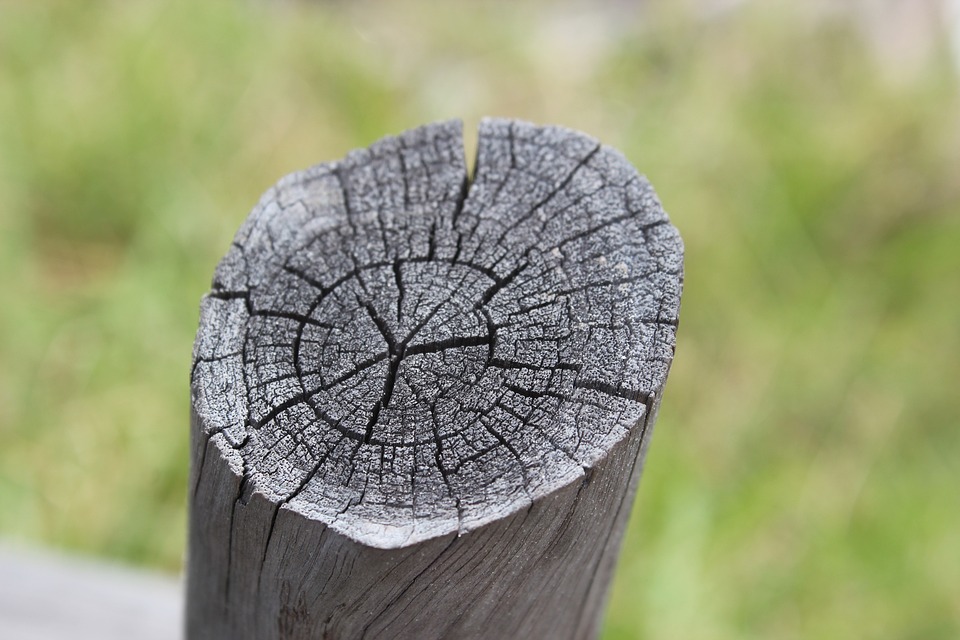
Python’s split() function is a powerful tool that allows for easy text manipulation and parsing. By splitting a string into a list of substrings based on a specified delimiter, developers can quickly extract and manipulate specific parts of text data with ease. This flexibility makes split() an invaluable tool for tasks such as data cleaning, text processing, and data analysis.
One of the key advantages of using split() is its simplicity and ease of use. The function takes a single argument, the delimiter on which to split the string, and returns a list of substrings. This makes it easy to quickly extract specific parts of a string without the need for complex regular expressions or other parsing techniques.
For example, suppose we have a string containing a list of names separated by commas:
“`
names = “Alice,Bob,Charlie”
“`
We can use split() to extract each individual name into a list:
“`
name_list = names.split(“,”)
print(name_list)
“`
Output:
“`
[‘Alice’, ‘Bob’, ‘Charlie’]
“`
This allows us to easily access and manipulate each individual name in the list, such as performing operations on each name separately or reformatting the names in a different way.
Split() can also be used to extract specific parts of a string based on more complex delimiters. For example, if we have a string containing a series of key-value pairs separated by colons:
“`
data = “name:Alice age:30 city:New York”
“`
We can use split() to extract the individual key-value pairs into a dictionary:
“`
data_dict = {}
for pair in data.split(” “):
key, value = pair.split(“:”)
data_dict[key] = value
print(data_dict)
“`
Output:
“`
{‘name’: ‘Alice’, ‘age’: ’30’, ‘city’: ‘New York’}
“`
This allows us to easily access and manipulate the individual key-value pairs in the dictionary, such as looking up the value associated with a specific key or adding new key-value pairs to the dictionary.
In addition to splitting strings based on a single delimiter, split() also supports splitting based on multiple delimiters. By passing a list of delimiters to the function, developers can split a string based on any one of the specified delimiters.
For example, suppose we have a string containing a list of names separated by commas, semicolons, or spaces:
“`
names = “Alice,Bob;Charlie Dave Faye”
“`
We can use split() to extract each individual name into a list, regardless of the delimiter used:
“`
name_list = names.split([“,”, “;”, ” “])
print(name_list)
“`
Output:
“`
[‘Alice’, ‘Bob’, ‘Charlie’, ‘Dave’, ‘Faye’]
“`
This flexibility allows developers to easily handle a wide range of text manipulation tasks without the need for complex parsing logic.
In conclusion, Python’s split() function is a powerful tool for text manipulation that offers flexibility, simplicity, and ease of use. By harnessing the capabilities of split(), developers can quickly and easily extract and manipulate specific parts of text data, making it an invaluable tool for a wide range of text processing tasks.