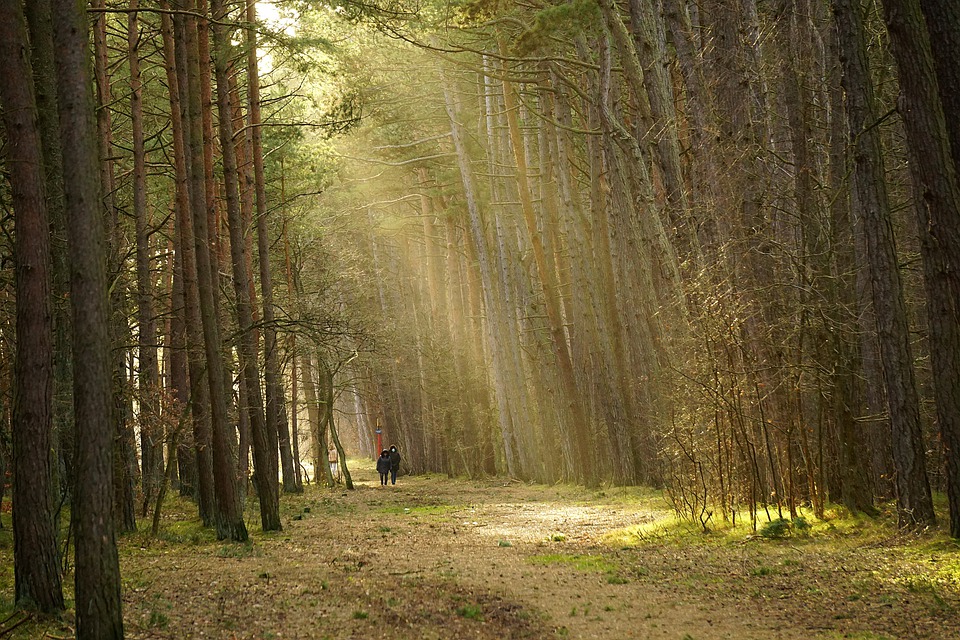
Go, also known as Golang, is a statically typed, compiled programming language developed by Google. It was created by Robert Griesemer, Rob Pike, and Ken Thompson in 2007 and has gained popularity for its simplicity and efficiency. In this article, we will explore the basics of Go programming language and then move on to more advanced concepts with code examples.
Basics of Go Programming Language
1. Hello World Program:
Let’s start with a classic “Hello World” program in Go:
“`go
package main
import “fmt”
func main() {
fmt.Println(“Hello, World!”)
}
“`
To run this program, save it in a file with a .go extension (e.g., hello.go) and run the following command in your terminal:
“`
go run hello.go
“`
This will print “Hello, World!” to the console.
2. Variables and Constants:
In Go, variables are declared using the `var` keyword followed by the variable name and type. Constants are declared using the `const` keyword. Here’s an example:
“`go
package main
import “fmt”
func main() {
var name string = “Alice”
const age = 30
fmt.Println(name, “is”, age, “years old”)
}
“`
3. Functions:
Functions in Go are declared using the `func` keyword. Here’s an example of a function that adds two numbers:
“`go
package main
import “fmt”
func add(a, b int) int {
return a + b
}
func main() {
result := add(5, 3)
fmt.Println(“Result:”, result)
}
“`
Advanced Concepts in Go Programming Language
1. Goroutines:
Goroutines are lightweight threads that allow concurrent execution in Go. They are created using the `go` keyword. Here’s an example of a simple goroutine:
“`go
package main
import (
“fmt”
“time”
)
func printNumbers() {
for i := 1; i