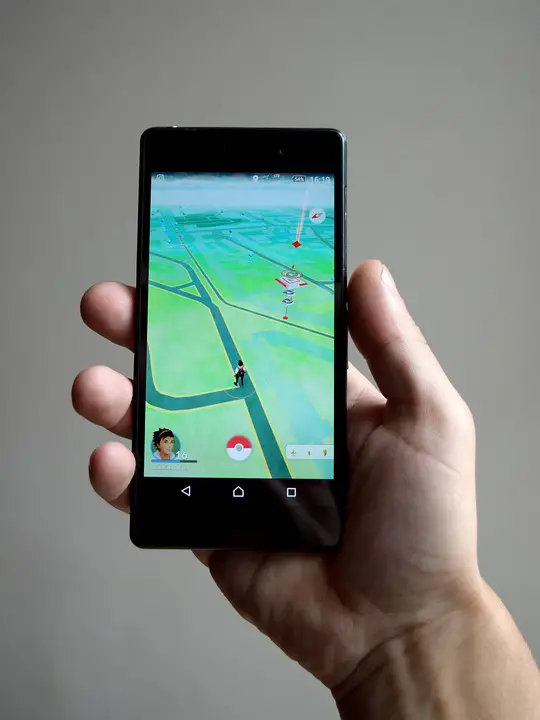
If you’re looking to dive into the world of programming, Go (also known as Golang) is a great language to start with. Developed by Google, Go is a statically typed, compiled language that is known for its simplicity, efficiency, and strong support for concurrency. In this beginner’s guide, we will walk you through the basics of Go programming and help you get started on your journey to becoming a proficient Go developer.
Setting Up Your Development Environment
Before you can start writing Go code, you’ll need to set up your development environment. The first step is to download and install the Go compiler and tools from the official Go website (https://golang.org/). Once you have Go installed on your machine, you can create a new directory for your Go projects and set up your workspace.
Writing Your First Go Program
Now that you have your development environment set up, it’s time to write your first Go program. Open your favorite text editor or integrated development environment (IDE) and create a new file with a .go extension. In this file, you can start writing your Go code.
Here’s a simple “Hello, World!” program in Go:
“`go
package main
import “fmt”
func main() {
fmt.Println(“Hello, World!”)
}
“`
Save this code in your file and run it using the Go compiler. You should see the output “Hello, World!” printed to your console. Congratulations, you’ve just written your first Go program!
Understanding Go Syntax
As you continue to learn Go, it’s important to familiarize yourself with the language’s syntax and conventions. Go is a statically typed language, which means that you must declare the type of variables before using them. Here’s an example of declaring and using variables in Go:
“`go
package main
import “fmt”
func main() {
var x int
x = 10
fmt.Println(x)
}
“`
In this code snippet, we declare a variable `x` of type `int` and assign it the value `10`. We then print the value of `x` to the console using the `fmt.Println()` function.
Working with Functions
Functions are a fundamental building block in Go programming. You can define your own functions to perform specific tasks or calculations in your program. Here’s an example of defining and using a function in Go:
“`go
package main
import “fmt”
func add(a, b int) int {
return a + b
}
func main() {
result := add(5, 3)
fmt.Println(result)
}
“`
In this code snippet, we define a function `add` that takes two integer parameters `a` and `b` and returns their sum. We then call the `add` function in the `main` function and print the result to the console.
Learning More About Go
As you continue to explore Go programming, there are many resources available to help you deepen your understanding of the language. The official Go documentation (https://golang.org/doc/) is a great place to start, as it provides comprehensive guides and tutorials on various aspects of Go programming. Additionally, there are numerous online courses, books, and forums dedicated to Go programming that can help you expand your knowledge and skills.
In conclusion, getting started with Go programming is an exciting journey that can lead to endless possibilities in software development. By familiarizing yourself with the basics of Go syntax, functions, and programming conventions, you’ll be well on your way to becoming a proficient Go developer. So grab your keyboard, fire up your editor, and start writing some Go code – the world of Go programming awaits!