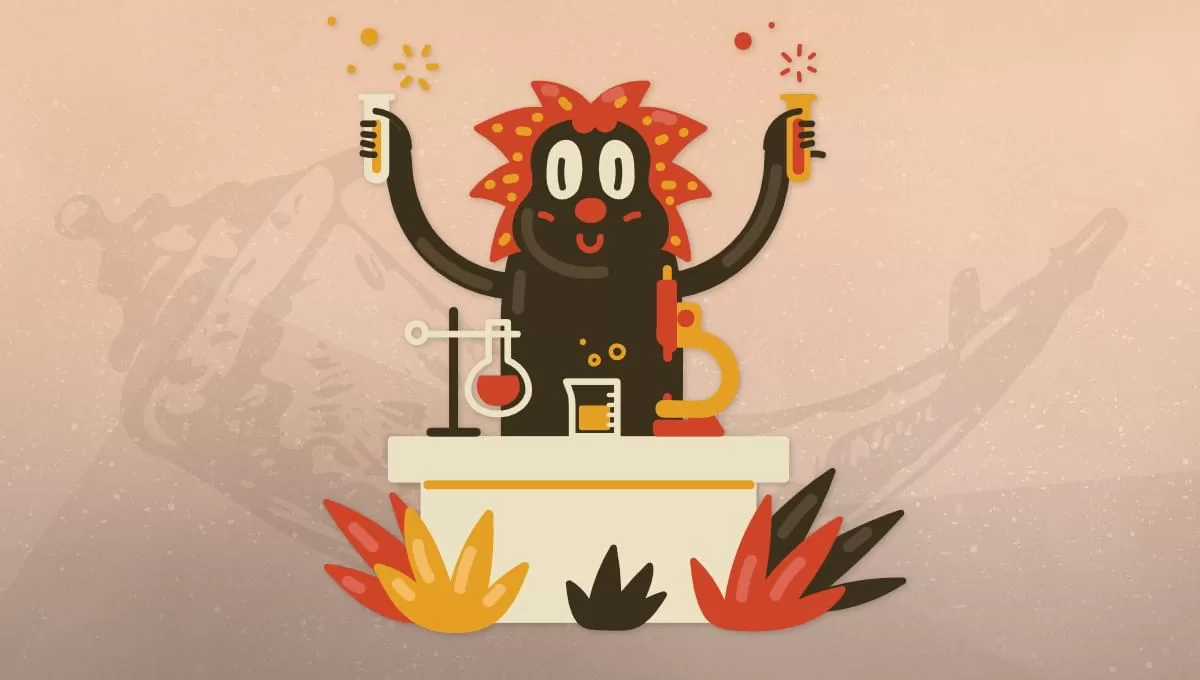
On this article, we’ll introduce Flask, a preferred microframework for Python that provides an alternative choice to the enormous that’s Django.
Flask is designed to be light-weight and versatile, permitting builders to create net apps rapidly and simply. On this article, we’ll cowl what Flask is, its key options, the advantages of its easy API, its flexibility in supporting third-party extensions, the most typical Flask extensions, and when is and isn’t a very good time to make use of Flask.
What Is Flask?
Flask is a micro net framework written in Python that’s used for growing net functions. It’s constructed on a easy philosophy of holding issues easy and light-weight, giving builders solely crucial instruments wanted to construct net apps with out pointless complexity.
It’s constructed on prime of the Werkzeug WSGI toolkit, which offers net server performance wanted for dealing with requests and responses, and the Jinja2 templating engine, which supplies Flask the flexibility to deal with HTML templates, permitting builders to create dynamic net functions.
Listed here are a number of the key options of Flask that make it such an ideal framework to work with:
- It has a easy API for creating net routes and dealing with requests.
- The Jinja templating engine provides assist for HTML templates, permitting builders to create net pages simply.
- It’s extremely extendable, resulting from its assist for third-party extensions, so a developer can set up extensions relying on their mission wants.
- It comes bundled with a growth server that makes it simple to check and debug apps.
Total, Flask offers a strong, versatile, but easy framework for constructing net functions. It’s a sensible choice for each new and skilled net builders, and is likely one of the hottest net frameworks within the Python net growth ecosystem.
Benefits of Flask
Let’s now take a extra detailed have a look at a number of the benefits of utilizing Flask in growth.
Simplicity. Flask’s design philosophy emphasizes simplicity, which makes it simple for builders at any stage to grasp and use. This additionally implies that builders have a really minimal studying curve, since they solely must study a couple of ideas and APIs to get began constructing net functions.
Flexibility. The micro nature of Flask — offering solely the core options of an online framework — offers builders the facility to customise and prolong it to go well with their necessities utilizing Flask extensions or third-party libraries.
Documentation. The Flask documentation could be very complete, with good protection of fundamental to superior matters, making it very simple for builders to learn to use the framework.
Compatibility. Flask is appropriate with a variety of Python variations, which makes it simple to make use of with present Python codebases. It additionally has assist for a number of net servers, which makes it simple to deploy it on a wide range of internet hosting platforms.
Fast growth. Flask’s simplicity and adaptability scale back the boilerplate wanted to arrange an utility, permitting builders to get began rapidly.
Across the Net, Flask is utilized in a number of attention-grabbing methods. A couple of notable examples are:
- PgAdmin. The Postgres admin interface runs on a Flask occasion, giving builders an interface the place they’ll handle their Postgres databases.
- Twilio. It is a communication platform that makes use of Flask in a number of of its APIs.
- Pinterest. This photo-sharing app makes use of Flask within the net growth stack, permitting its staff to create some customized options and integrations simply.
When to Use Flask
Flask’s simplicity and ease of use make it a superb alternative for a variety of net initiatives:
- Prototyping. Its ease of use and adaptability make it a superb alternative for rapidly creating prototypes permitting builders to construct and take a look at new options quick.
- Creating RESTful APIs. Its personal easy API makes it simple to create and deal with HTTP requests.
- Ecommerce apps. It really works properly for constructing on-line marketplaces and ecommerce platforms.
- Finance. It’s helpful for constructing monetary functions, with account administration, transaction processing, and funding monitoring.
- AI. It provides a helpful and simple technique to construct and deploy AI coaching fashions.
When Not To Use Flask
Whereas Flask is a superb framework and has an a variety of benefits and nice options, there are conditions these options work towards it. Let’s discover a number of the initiatives that go well with different forms of frameworks higher.
Tasks that require built-in performance. Being a microframework, Flask solely offers the core bits wanted to create an online utility. If a mission requires, say, an admin interface, authentication, or an ORM, then Django is a greater possibility.
Tasks with strict safety necessities. As Flask is a versatile framework, we’ve to depend on third-party extensions to implement some stage of safety in our apps. Whereas this definitely works, it’s higher to depend on a extra battle-tested framework that takes a safer strategy, reminiscent of Twister or Twisted.
Tasks that implement some coding customary. As a result of Flask’s flexibility, growing functions on it leaves builders to make apps in any means they see match. Nonetheless, frameworks like Django be certain that builders comply with a specific conference, that means that builders can transfer from one mission to different simply.
Setting Up a Flask Improvement Setting
Let’s now have a look at how one can get began with Flask, from organising the event setting, to set up, and eventually spinning up a minimal utility.
Stipulations
Python needs to be put in on the event machine. Listed here are directions for that (though we could have already got it put in).
Create a Digital Setting
A digital setting is an remoted Python setting the place we will set up packages for a given mission with out affecting the worldwide Python set up. (Right here’s additional dialogue about why digital environments are helpful.) There are totally different packages for creating digital environments in Python, reminiscent of virtualenv, venv, or Conda.
On this article, we’ll use virtualenv
. We are able to set up it with the next command:
<code class="bash language-bash">pip <span class="token function">set up</span> virtualenv </code>
As soon as virtualenv
has been put in, we will create a listing the place our Flask utility will reside. We are able to name the listing no matter we would like — apart from Flask
, as that may trigger conflicts. We’ll name ours flask_intro
:
<code class="bash language-bash"><span class="token function">mkdir</span> flask_intro </code>
Subsequent, become that listing in order that we will begin utilizing it:
<code class="bash language-bash"><span class="token builtin class-name">cd</span> flask_intro </code>
In that listing, let’s now create our digital setting, utilizing the next command:
<code class="bash language-bash">virtualenv myenv </code>
The command above creates a digital setting and calls it myenv
. Let’s activate it in order that we will set up Flask in it. To activate the digital setting on Linux or macOS, use the next command:
<code class="bash language-bash"><span class="token builtin class-name">.</span> myenv/bin/activate </code>
On Home windows, use this command:
<code class="bash language-bash"><span class="token builtin class-name">.</span> myenv<span class="token punctuation">\</span>Scripts<span class="token punctuation">\</span>activate </code>
As soon as our digital setting has been activated, it can present the title of the digital setting on the shell immediate, much like the output under:
<code class="bash language-bash"><span class="token punctuation">(</span>myenv<span class="token punctuation">)</span>/~<span class="token punctuation">(</span>path to your mission listing<span class="token punctuation">)</span>$ </code>
Inside our activated digital setting, we will go forward and set up Flask, utilizing the next command:
<code class="bash language-bash">pip <span class="token function">set up</span> Flask </code>
As soon as Flask finishes putting in, let’s go forward and create a minimal utility. We’ll create a module that may maintain our Flask utility. For simplicity’s sake, let’s name it whats up.py
. On Linux or macOS we will use the next command to create the file in our flask_intro
listing:
<code class="bash language-bash"><span class="token function">contact</span> whats up.py </code>
The command above creates a file named whats up.py
. We may additionally use a growth setting to create the file. As soon as the file has been created, put the under code in it and reserve it:
<code class=" <a href="https://yourselfhood.com/how-to-write-efficient-python-code-a-tutorial-for-beginners/" class="lar_link" data-linkid="2205" data-postid="1846" title="Python" target="_blank" >python</a> language- <a href="https://yourselfhood.com/how-to-write-efficient-python-code-a-tutorial-for-beginners/" class="lar_link" data-linkid="2205" data-postid="1846" title="Python" target="_blank" >python</a>"> <span class="token keyword">from</span> flask <span class="token keyword">import</span> Flask app <span class="token operator">=</span> Flask<span class="token punctuation">(</span>__name__<span class="token punctuation">)</span> <span class="token decorator annotation punctuation">@app<span class="token punctuation">.</span>route</span><span class="token punctuation">(</span><span class="token string">"https://www.sitepoint.com/"</span><span class="token punctuation">)</span> <span class="token keyword">def</span> <span class="token function">hello_world</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">:</span> <span class="token keyword">return</span> <span class="token string">'Hi there, World!'</span> </code>
Within the code above, we import the Flask
class from the flask
module, then create an occasion of Flask
that we name app
and cross the __name__
variable.
Then we’ve the route decorator @app.route(“\”)
, which implies that the hello_world()
perform might be triggered when somebody visits the foundation route of our utility as soon as it’s run.
There are numerous ways in which we may run the appliance, so let’s have a look at a few of them. The primary means is to provide the flask
command with a variety of arguments: --app
, then the title of the module that holds, our Flask utility, after which run
. See under:
<code class="bash language-bash">flask –app <span class="token operator"><</span>the title of your module<span class="token operator">></span> run </code>
Utilizing the instance above to run our pattern utility:
<code class="bash language-bash">flask –app whats up run </code>
That may run our utility on the default port 5000
so the appliance might be out there on http://localhost:5000/
or at http://127.0.0.1:5000/
. If we would like the appliance to be out there on a special port, we will specify the port utilizing -p
or --port
possibility. For instance:
<code class="bash language-bash">flask --app whats up run --port<span class="token operator">=</span><span class="token number">8080</span> </code>
That may run the server on port 8080. The opposite means we will run the appliance is by simply utilizing the flask run
instructions. Nonetheless, for us to have the ability to do this, we have to inform Flask the title of the module that may maintain the Flask occasion, and we do this by setting the FLASK_APP
setting variable. So in our case, the Flask utility is contained in a file named whats up.py
. So we will set it this fashion:
<code class="bash language-bash"><span class="token builtin class-name">export</span> <span class="token assign-left variable">FLASK_APP</span><span class="token operator">=</span>whats up </code>
Now that we’ve set the FLASK_APP
setting variable, we will run the event server like so:
<code class="bash language-bash">flask run </code>
With that code, we now have an online utility working. This demonstrates the core philosophy of Flask: we don’t want a number of boilerplate code to get issues going. Nonetheless, the appliance we’ve arrange above isn’t very useful or helpful, because it solely renders the string “Hi there World!” on our net web page. To do something extra helpful, we will flip to templates. We’ll have a look at how one can deal with them subsequent.
Flask Templates
Flask templates are a technique to create dynamic net pages that may show totally different content material based mostly on varied elements, reminiscent of knowledge from a database, or consumer enter. Templates in Flask are a mix of HTML and particular placeholders referred to as template variables which can be changed with precise values at runtime.
Templates are saved within the templates
listing. So to make use of templates, we have to import the render_template()
methodology from flask
. The render_template()
methodology takes a template title and any optionally available knowledge that must be handed to the template.
Let’s see an instance of a perform that makes use of a template to render an online web page:
<code class=" <a href="https://yourselfhood.com/how-to-write-efficient-python-code-a-tutorial-for-beginners/" class="lar_link" data-linkid="2205" data-postid="1846" title="Python" target="_blank" >python</a> language- <a href="https://yourselfhood.com/how-to-write-efficient-python-code-a-tutorial-for-beginners/" class="lar_link" data-linkid="2205" data-postid="1846" title="Python" target="_blank" >python</a>"> <span class="token keyword">from</span> flask <span class="token keyword">import</span> Flask<span class="token punctuation">,</span> render_template app <span class="token operator">=</span> Flask<span class="token punctuation">(</span>__name__<span class="token punctuation">)</span> <span class="token decorator annotation punctuation">@app<span class="token punctuation">.</span>route</span><span class="token punctuation">(</span><span class="token string">"https://www.sitepoint.com/"</span><span class="token punctuation">)</span> <span class="token keyword">def</span> <span class="token function">index</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">:</span> title <span class="token operator">=</span> <span class="token string">'Welcome to my web site!'</span> message <span class="token operator">=</span> <span class="token string">'That is an instance of utilizing Flask templates.'</span> <span class="token keyword">return</span> render_template<span class="token punctuation">(</span><span class="token string">'index.html'</span><span class="token punctuation">,</span> title<span class="token operator">=</span>title<span class="token punctuation">,</span> message<span class="token operator">=</span>message<span class="token punctuation">)</span> </code>
Within the instance above, we’ve a view perform — index()
— that’s sure to the foundation URL (“/”) by the @app.route()
decorator. The perform has two variables, title
and message
. Lastly, we cross the template index.html
to the render_template()
, along with the title
and message
variables.
For the code above to work, we have to have an index.html
template residing in a templates listing. So the template will look one thing like this:
<code class="markup language-markup"># index.html <span class="token doctype"><span class="token punctuation"><!</span><span class="token name">doctype</span> <span class="token name">html</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>html</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>head</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>title</span><span class="token punctuation">></span></span>{{ title }}<span class="token tag"><span class="token tag"><span class="token punctuation"></</span>title</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"></</span>head</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>physique</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>h1</span><span class="token punctuation">></span></span>{{ title }}<span class="token tag"><span class="token tag"><span class="token punctuation"></</span>h1</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>p</span><span class="token punctuation">></span></span>{{ message }}<span class="token tag"><span class="token tag"><span class="token punctuation"></</span>p</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"></</span>physique</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"></</span>html</span><span class="token punctuation">></span></span> </code>
Within the index.html
file, the placeholders {{title}}
and {{ message }}
are changed with the values handed to the template within the render_template()
methodology.
Templates may embody extra complicated logic reminiscent of if
statements and for
loops, which permit for extra dynamic pages to be generated.
So templates in Flask present builders with a really highly effective possibility for creating dynamic net pages wealthy with user-generate data.
Flask Routing
Most net functions could have a couple of URL, so we have to have a means of figuring out which perform handles which URL. In Flask, this mapping is called routing — the method of binding or mapping URLs to view features. Binding URLs to view features permits the appliance to deal with several types of requests, reminiscent of GET
, POST
, PUT
, DELETE
, and extra. It additionally permits the appliance to deal with a number of requests from totally different purchasers.
To arrange routes in Flask, we use the route()
decorator. The decorator binds a URL to a view perform — so when a consumer visits a URL that exists on our utility, Flask triggers the related view perform to deal with the request.
Let’s see an instance:
<code class=" <a href="https://yourselfhood.com/how-to-write-efficient-python-code-a-tutorial-for-beginners/" class="lar_link" data-linkid="2205" data-postid="1846" title="Python" target="_blank" >python</a> language- <a href="https://yourselfhood.com/how-to-write-efficient-python-code-a-tutorial-for-beginners/" class="lar_link" data-linkid="2205" data-postid="1846" title="Python" target="_blank" >python</a>"> <span class="token keyword">from</span> flask <span class="token keyword">import</span> Flask<span class="token punctuation">,</span> render_template app <span class="token operator">=</span> Flask<span class="token punctuation">(</span>__name__<span class="token punctuation">)</span> <span class="token decorator annotation punctuation">@app<span class="token punctuation">.</span>route</span><span class="token punctuation">(</span><span class="token string">"/about"</span><span class="token punctuation">)</span> <span class="token keyword">def</span> <span class="token function">about</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">:</span> <span class="token keyword">return</span> <span class="token string">"That is the about web page"</span> </code>
Within the instance above, we outline an about URL (/about
). When the appliance receives a request for the about
URL, Flask calls the about()
perform, which returns the string “That is the about web page”.
To date, regardless that these examples return totally different pages, all of them simply use the GET
HTTP request. So as to have the ability to deal with any particular request, we will specify the HTTP methodology as an optionally available argument to the route()
decorator.
Let’s see an instance of a PUT
request:
<code class=" <a href="https://yourselfhood.com/how-to-write-efficient-python-code-a-tutorial-for-beginners/" class="lar_link" data-linkid="2205" data-postid="1846" title="Python" target="_blank" >python</a> language- <a href="https://yourselfhood.com/how-to-write-efficient-python-code-a-tutorial-for-beginners/" class="lar_link" data-linkid="2205" data-postid="1846" title="Python" target="_blank" >python</a>"><span class="token keyword">from</span> flask <span class="token keyword">import</span> Flask<span class="token punctuation">,</span> request app <span class="token operator">=</span> Flask<span class="token punctuation">(</span>__name__<span class="token punctuation">)</span> <span class="token decorator annotation punctuation">@app<span class="token punctuation">.</span>route</span><span class="token punctuation">(</span><span class="token string">'/customers/<int:user_id>'</span><span class="token punctuation">,</span> <a href="https://yourselfhood.com/how-to-compensate-for-blind-spots-and-biases-in-your-security-strategy/">strategies</a><span class="token operator">=</span><span class="token punctuation">[</span><span class="token string">'PUT'</span><span class="token punctuation">]</span><span class="token punctuation">)</span> <span class="token keyword">def</span> <span class="token function">update_user</span><span class="token punctuation">(</span>user_id<span class="token punctuation">)</span><span class="token punctuation">:</span> knowledge <span class="token operator">=</span> request<span class="token punctuation">.</span>get_json<span class="token punctuation">(</span><span class="token punctuation">)</span> <span class="token keyword">return</span> <span class="token punctuation">{</span><span class="token string">'message'</span><span class="token punctuation">:</span> <span class="token string-interpolation"><span class="token string">f'Person </span><span class="token interpolation"><span class="token punctuation">{</span>user_id<span class="token punctuation">}</span></span><span class="token string"> up to date efficiently'</span></span><span class="token punctuation">}</span><span class="token punctuation">,</span> <span class="token number">200</span> </code>
On this instance, we outline a route that handles a PUT
request to replace consumer particulars given their user_id
. We use
within the route to point that the consumer ID ought to be an integer.
Within the update_user()
perform, we get the consumer knowledge from the request physique utilizing the request.get_json()
methodology. We do one thing with the consumer knowledge, reminiscent of updating the consumer within the database, after which return a response indicating success or failure together with an HTTP standing code (a 200
on this case to indicate success).
Total, routing permits Flask to have the ability to deal with several types of requests and permits our utility to deal with and act on knowledge in another way, relying on the URL {that a} consumer visits.
Flask Varieties and Validation
Aside from displaying knowledge for customers, Flask templates may take enter from customers for additional processing or storage. For that, Flask offers built-in assist for processing HTML varieties and dealing with consumer enter. Flask varieties are based mostly on the WTForms library, which offers a versatile and highly effective technique to deal with type knowledge and carry out validations. Nonetheless, the library isn’t part of the usual Flask set up, so we have to set up it utilizing the next command:
<code class="bash language-bash">pip <span class="token function">set up</span> WTForms </code>
As soon as we’ve put in WTForms, to make use of varieties in Flask we have to outline a type class that may inherit from flask_wtf.FlaskForm
. The category will comprise the fields which can be going to be on the shape and any validation guidelines that ought to be utilized to them.
Let’s see an instance of a login type:
<code class=" <a href="https://yourselfhood.com/how-to-write-efficient-python-code-a-tutorial-for-beginners/" class="lar_link" data-linkid="2205" data-postid="1846" title="Python" target="_blank" >python</a> language- <a href="https://yourselfhood.com/how-to-write-efficient-python-code-a-tutorial-for-beginners/" class="lar_link" data-linkid="2205" data-postid="1846" title="Python" target="_blank" >python</a>"> <span class="token keyword">from</span> flask_wtf <span class="token keyword">import</span> FlaskForm <span class="token keyword">from</span> wtforms <span class="token keyword">import</span> StringField<span class="token punctuation">,</span> PasswordField<span class="token punctuation">,</span> SubmitField <span class="token keyword">from</span> wtforms<span class="token punctuation">.</span>validators <span class="token keyword">import</span> DataRequired<span class="token punctuation">,</span> E mail<span class="token punctuation">,</span> Size <span class="token keyword">class</span> <span class="token class-name">LoginForm</span><span class="token punctuation">(</span>FlaskForm<span class="token punctuation">)</span><span class="token punctuation">:</span> e-mail <span class="token operator">=</span> StringField<span class="token punctuation">(</span><span class="token string">'E mail'</span><span class="token punctuation">,</span> validators<span class="token operator">=</span><span class="token punctuation">[</span>DataRequired<span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">,</span> Email<span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">]</span><span class="token punctuation">)</span> password <span class="token operator">=</span> PasswordField<span class="token punctuation">(</span><span class="token string">'Password'</span><span class="token punctuation">,</span> validators<span class="token operator">=</span><span class="token punctuation">[</span>DataRequired<span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">,</span> Length<span class="token punctuation">(</span><span class="token builtin">min</span><span class="token operator">=</span><span class="token number">6</span><span class="token punctuation">)</span><span class="token punctuation">]</span><span class="token punctuation">)</span> submit <span class="token operator">=</span> SubmitField<span class="token punctuation">(</span><span class="token string">'Log In'</span><span class="token punctuation">)</span> </code>
Within the instance above, we outline a login type with two fields — e-mail
and password
— and a submit button. We even have a validators
argument that’s used to specify validation guidelines for every subject. For instance, on this case we require the e-mail
subject to comprise a sound e-mail deal with and the password
subject to comprise a password of no fewer than six characters.
As soon as we’ve outlined the shape class, we will use it within the login view perform to render the shape and course of the shape knowledge submitted by the consumer. Let’s see an instance of the view perform:
<code class=" <a href="https://yourselfhood.com/how-to-write-efficient-python-code-a-tutorial-for-beginners/" class="lar_link" data-linkid="2205" data-postid="1846" title="Python" target="_blank" >python</a> language- <a href="https://yourselfhood.com/how-to-write-efficient-python-code-a-tutorial-for-beginners/" class="lar_link" data-linkid="2205" data-postid="1846" title="Python" target="_blank" >python</a>"> <span class="token keyword">from</span> flask <span class="token keyword">import</span> render_template<span class="token punctuation">,</span> request <span class="token keyword">from</span> <span class="token punctuation">.</span>varieties <span class="token keyword">import</span> LoginForm <span class="token decorator annotation punctuation">@app<span class="token punctuation">.</span>route</span><span class="token punctuation">(</span><span class="token string">'/login'</span><span class="token punctuation">,</span> strategies<span class="token operator">=</span><span class="token punctuation">[</span><span class="token string">'GET'</span><span class="token punctuation">,</span> <span class="token string">'POST'</span><span class="token punctuation">]</span><span class="token punctuation">)</span> <span class="token keyword">def</span> <span class="token function">login</span><span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">:</span> type <span class="token operator">=</span> LoginForm<span class="token punctuation">(</span><span class="token punctuation">)</span> <span class="token keyword">if</span> type<span class="token punctuation">.</span>validate_on_submit<span class="token punctuation">(</span><span class="token punctuation">)</span><span class="token punctuation">:</span> e-mail <span class="token operator">=</span> type<span class="token punctuation">.</span>e-mail<span class="token punctuation">.</span>knowledge password <span class="token operator">=</span> type<span class="token punctuation">.</span>password<span class="token punctuation">.</span>knowledge <span class="token keyword">return</span> render_template<span class="token punctuation">(</span><span class="token string">'login.html'</span><span class="token punctuation">,</span> type<span class="token operator">=</span>type<span class="token punctuation">)</span> </code>
Within the instance above, we’ve a login
view that accepts two HTTP strategies (GET
and POST
), so when customers entry the URL from a browser the LoginForm
is rendered as an HTML type utilizing the render_template
methodology, and when a consumer submits the shape we test if the shape is legitimate utilizing the validate_on_submit
methodology. If the shape is legitimate, we entry the e-mail and password.
The login.html
type may look one thing like this:
<code class="markup language-markup"># login.html <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>h1</span><span class="token punctuation">></span></span>Login<span class="token tag"><span class="token tag"><span class="token punctuation"></</span>h1</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>type</span> <span class="token attr-name">methodology</span><span class="token attr-value"><span class="token punctuation attr-equals">=</span><span class="token punctuation">"</span>POST<span class="token punctuation">"</span></span><span class="token punctuation">></span></span> { type.csrf_token } <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>div</span><span class="token punctuation">></span></span> { type.e-mail.label } {} <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>span</span> <span class="token style-attr"><span class="token attr-name">model</span><span class="token attr-value"><span class="token punctuation attr-equals">=</span><span class="token punctuation">"</span><span class="token style language-css"><span class="token property">colour</span><span class="token punctuation">:</span> <span class="token color">crimson</span><span class="token punctuation">;</span></span><span class="token punctuation">"</span></span></span><span class="token punctuation">></span></span>[{{ error }}]<span class="token tag"><span class="token tag"><span class="token punctuation"></</span>span</span><span class="token punctuation">></span></span> {% endfor %} <span class="token tag"><span class="token tag"><span class="token punctuation"></</span>div</span><span class="token punctuation">></span></span> <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>div</span><span class="token punctuation">></span></span> { type.password.label } { type.password() } % for error in type.password.errors % <span class="token tag"><span class="token tag"><span class="token punctuation"><</span>span</span> <span class="token style-attr"><span class="token attr-name">model</span><span class="token attr-value"><span class="token punctuation attr-equals">=</span><span class="token punctuation">"</span><span class="token style language-css"><span class="token property">colour</span><span class="token punctuation">:</span> <span class="token color">crimson</span><span class="token punctuation">;</span></span><span class="token punctuation">"</span></span></span><span class="token punctuation">></span></span>[{{ error }}]<span class="token tag"><span class="token tag"><span class="token punctuation"></</span>span</span><span class="token punctuation">></span></span> {% endfor %} <span class="token tag"><span class="token tag"><span class="token punctuation"></</span>div</span><span class="token punctuation">></span></span> { type.submit() } <span class="token tag"><span class="token tag"><span class="token punctuation"></</span>type</span><span class="token punctuation">></span></span> </code>
The above template will render the e-mail
and password
fields, together with their labels, and a submit button with the textual content “Login”. The type.csrf_token
subject is included to forestall cross-site request forgery (CSRF) assaults. The {% for %}
loops are used to show any validation errors which will happen.
Through the use of Flask varieties, we’ve a strong means of dealing with consumer enter, and we’ll be capable to validate the info they enter.
Flask Extensions
As we’ve seen, Flask is a microframework that solely contains crucial elements wanted to create an online utility. Nonetheless, if we have to add performance that isn’t supplied out of the field by Flask, we have to add packages to the set up. Flask extensions are the way in which we offer this extra performance. We are able to merely set up the bundle we’d like. There are a lot of extensions made by the Flask group.
Listed here are a number of the hottest ones:
- Flask-SQLAlchemy: offers integration with the SQLAlchemy toolkit that makes it simple to work together with databases.
- Flask-Login: offers consumer authentication and session administration to Flask.
- Flask-Mail: offers a easy interface to ship emails from Flask.
There are tons of of extensions made by the Flask group to deal with totally different performance. Utilizing the extensions is mostly simple. First, we have to set up the extension we would like utilizing pip.
Let’s see an instance of utilizing Flask-SQLAlchemy. First, we have to set up it:
<code class="bash language-bash">pip <span class="token function">set up</span> flask-sqlalchemy </code>
Subsequent, we have to configure it. For instance:
<code class=" <a href="https://yourselfhood.com/how-to-write-efficient-python-code-a-tutorial-for-beginners/" class="lar_link" data-linkid="2205" data-postid="1846" title="Python" target="_blank" >python</a> language- <a href="https://yourselfhood.com/how-to-write-efficient-python-code-a-tutorial-for-beginners/" class="lar_link" data-linkid="2205" data-postid="1846" title="Python" target="_blank" >python</a>"> <span class="token keyword">from</span> flask <span class="token keyword">import</span> Flask <span class="token keyword">from</span> flask_sqlalchemy <span class="token keyword">import</span> SQLAlchemy app <span class="token operator">=</span> Flask<span class="token punctuation">(</span>__name__<span class="token punctuation">)</span> app<span class="token punctuation">.</span>config<span class="token punctuation">[</span><span class="token string">'SQLALCHEMY_DATABASE_URI'</span><span class="token punctuation">]</span> <span class="token operator">=</span> <span class="token string">'sqlite:///instance.db'</span> db <span class="token operator">=</span> SQLAlchemy<span class="token punctuation">(</span>app<span class="token punctuation">)</span> <span class="token keyword">class</span> <span class="token class-name">Person</span><span class="token punctuation">(</span>db<span class="token punctuation">.</span>Mannequin<span class="token punctuation">)</span><span class="token punctuation">:</span> <span class="token builtin">id</span> <span class="token operator">=</span> db<span class="token punctuation">.</span>Column<span class="token punctuation">(</span>db<span class="token punctuation">.</span>Integer<span class="token punctuation">,</span> primary_key<span class="token operator">=</span><span class="token boolean">True</span><span class="token punctuation">)</span> username <span class="token operator">=</span> db<span class="token punctuation">.</span>Column<span class="token punctuation">(</span>db<span class="token punctuation">.</span>String<span class="token punctuation">(</span><span class="token number">80</span><span class="token punctuation">)</span><span class="token punctuation">,</span> distinctive<span class="token operator">=</span><span class="token boolean">True</span><span class="token punctuation">,</span> nullable<span class="token operator">=</span><span class="token boolean">False</span><span class="token punctuation">)</span> e-mail <span class="token operator">=</span> db<span class="token punctuation">.</span>Column<span class="token punctuation">(</span>db<span class="token punctuation">.</span>String<span class="token punctuation">(</span><span class="token number">120</span><span class="token punctuation">)</span><span class="token punctuation">,</span> distinctive<span class="token operator">=</span><span class="token boolean">True</span><span class="token punctuation">,</span> nullable<span class="token operator">=</span><span class="token boolean">False</span><span class="token punctuation">)</span> <span class="token keyword">def</span> <span class="token function">__repr__</span><span class="token punctuation">(</span>self<span class="token punctuation">)</span><span class="token punctuation">:</span> <span class="token keyword">return</span> <span class="token string">'<Person %r>'</span> <span class="token operator">%</span> self<span class="token punctuation">.</span>username </code>
Within the instance above, we’ve a Person
mannequin with a username
and e-mail
subject. We additionally configure SQLALCHEMY_DATABASE_URI
, indicating that we’re utilizing an SQLite database situated at instance.db
. With that set, we now have entry to the db
object that permits us to work together with the database. For instance, we may create a brand new consumer and add it to the database, like so:
<code class=" <a href="https://yourselfhood.com/how-to-write-efficient-python-code-a-tutorial-for-beginners/" class="lar_link" data-linkid="2205" data-postid="1846" title="Python" target="_blank" >python</a> language- <a href="https://yourselfhood.com/how-to-write-efficient-python-code-a-tutorial-for-beginners/" class="lar_link" data-linkid="2205" data-postid="1846" title="Python" target="_blank" >python</a>"> <span class="token keyword">from</span> app <span class="token keyword">import</span> db<span class="token punctuation">,</span> Person consumer <span class="token operator">=</span> Person<span class="token punctuation">(</span>username<span class="token operator">=</span><span class="token string">'john'</span><span class="token punctuation">,</span> e-mail<span class="token operator">=</span><span class="token string">'john@instance.com'</span><span class="token punctuation">)</span> db<span class="token punctuation">.</span>session<span class="token punctuation">.</span>add<span class="token punctuation">(</span>consumer<span class="token punctuation">)</span> db<span class="token punctuation">.</span>session<span class="token punctuation">.</span>commit<span class="token punctuation">(</span><span class="token punctuation">)</span> </code>
With Flask extensions, our utility is ready to have extra performance than it could usually have with the core Flask implementation.
Conclusion
On this article, we launched Flask, a light-weight and versatile net framework for Python. We mentioned some great benefits of utilizing Flask for net growth, together with its simplicity, flexibility, and ease of use. We additionally lined how one can arrange a growth setting, create routes, use templates, deal with varieties, and use extensions like Flask-SQLAlchemy.
To summarize, Flask is a superb alternative for constructing net functions of any measurement, from small private initiatives to large-scale industrial functions. It’s simple to study and use, but in addition provides superior options by means of its many extensions.
If you happen to’re excited about studying extra about Flask, listed here are some further assets:
If you happen to’d wish to study extra about Django and Flask and their finest use instances, take a look at Python Net Improvement with Django and Flask.