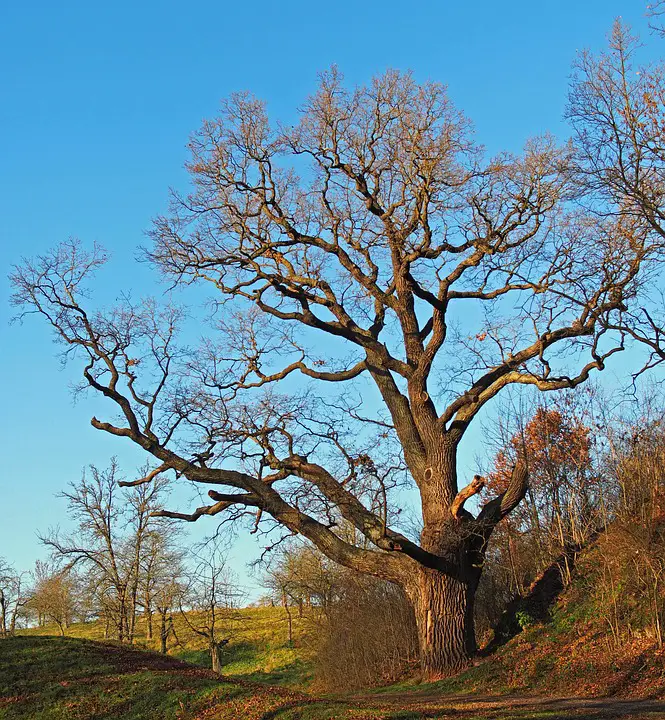
Python’s split() method is a powerful tool that allows you to break a string into a list of substrings based on a specified delimiter. While this method is commonly used for simple text processing tasks, there are many innovative techniques you can try to get creative with split() and enhance your programming skills. In this article, we will explore some of these techniques and provide examples of how you can use split() in unique ways.
1. Splitting a String into Characters
One interesting way to use split() is to break a string into individual characters. By setting an empty string as the delimiter, you can achieve this easily. Here’s an example:
“` python
text = “Hello, World!”
characters = text.split(”)
print(characters)
“`
This will output: [‘H’, ‘e’, ‘l’, ‘l’, ‘o’, ‘,’, ‘ ‘, ‘W’, ‘o’, ‘r’, ‘l’, ‘d’, ‘!’]
2. Splitting a String into Sentences
If you have a block of text and want to split it into sentences, you can use split() with a period as the delimiter. Here’s an example:
“` python
text = “ Python is a versatile programming language. It is used for web development, data analysis, and more.”
sentences = text.split(‘.’)
print(sentences)
“`
This will output: [‘ Python is a versatile programming language’, ‘ It is used for web development, data analysis, and more’, ”]
3. Splitting a String into Words
Splitting a string into words is a common use case for split(). You can achieve this by using a space as the delimiter. Here’s an example:
“` python
text = “Get creative with Python split”
words = text.split(‘ ‘)
print(words)
“`
This will output: [‘Get’, ‘creative’, ‘with’, ‘ Python’, ‘split’]
4. Splitting a String into Lines
If you have multi-line text and want to split it into individual lines, you can use split() with a newline character as the delimiter. Here’s an example:
“` python
text = “ Python\nis\na\nversatile\nprogramming\nlanguage”
lines = text.split(‘\n’)
print(lines)
“`
This will output: [‘ Python’, ‘is’, ‘a’, ‘versatile’, ‘programming’, ‘language’]
5. Splitting a String into Key-Value Pairs
You can also split a string into key-value pairs by using a specific delimiter. For example, if you have a string with pairs separated by a colon, you can split it like this:
“` python
text = “name:John age:30 city:New York”
pairs = text.split(‘ ‘)
key_value_pairs = {}
for pair in pairs:
key, value = pair.split(‘:’)
key_value_pairs[key] = value
print(key_value_pairs)
“`
This will output: {‘name’: ‘John’, ‘age’: ’30’, ‘city’: ‘New York’}
Overall, there are countless ways to get creative with Python’s split() method. By experimenting with different delimiters and combining split() with other string manipulation techniques, you can unlock new possibilities and enhance your programming skills. So go ahead and try out these innovative techniques to see what you can achieve with split() in Python!