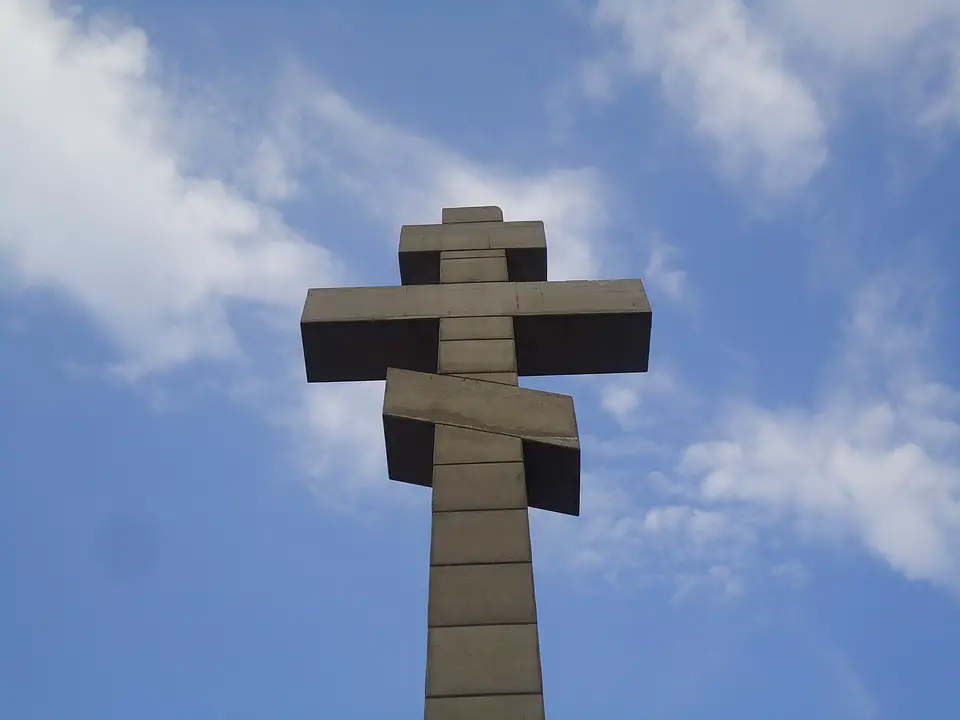
Python is undoubtedly one of the most popular programming languages in the world. Known for its simplicity and readability, Python is widely used in various fields such as web development, data science, machine learning, and more. While many programmers are familiar with the basic features of Python, there are some advanced concepts that can take your coding skills to the next level. One such concept is Python sets.
Sets in Python are an unordered collection of unique elements. They are similar to lists or dictionaries, but with some key differences that make them extremely useful in certain situations. In this article, we will explore the basics of Python sets and then delve into some advanced techniques that can help you elevate your coding game.
Basics of Python Sets
To create a set in Python, you can use curly braces {} or the set() function. Sets can contain any immutable data type such as integers, floats, strings, and tuples. Here is an example of how to create a set in Python:
“` python
my_set = {1, 2, 3, 4, 5}
“`
Unlike lists, sets do not allow duplicate elements. If you try to add a duplicate element to a set, it will simply be ignored. Sets are also unordered, which means that the elements are not stored in any particular order.
One of the main advantages of using sets in Python is their efficiency in performing set operations such as union, intersection, difference, and symmetric difference. These operations can be performed using built-in methods such as union(), intersection(), difference(), and symmetric_difference(). Here is an example of how to perform a union operation on two sets:
“` python
set1 = {1, 2, 3}
set2 = {3, 4, 5}
union_set = set1.union(set2)
print(union_set)
“`
Advanced Techniques with Python Sets
Now that you are familiar with the basics of Python sets, let’s explore some advanced techniques that can help you take your coding skills to the next level.
1. Set Comprehensions: Similar to list comprehensions, set comprehensions allow you to create sets using a concise syntax. Here is an example of how to create a set using set comprehension:
“` python
my_set = {x for x in range(10) if x % 2 == 0}
“`
2. Frozensets: Frozensets are immutable sets that cannot be changed once they are created. They can be used as dictionary keys or elements of other sets. Here is an example of how to create a frozenset in Python:
“` python
my_frozenset = frozenset([1, 2, 3, 4, 5])
“`
3. Set Operations: Python sets support a variety of set operations that can help you manipulate sets in different ways. Some of the most common set operations include union, intersection, difference, and symmetric difference.
By mastering these advanced techniques with Python sets, you can streamline your code, improve performance, and write more efficient and readable code. Whether you are working on a data science project, a web application, or a machine learning algorithm, understanding how to leverage Python sets can help you elevate your coding game to new heights.
In conclusion, Python sets are a powerful and versatile data structure that can help you write more efficient and concise code. By mastering the basics of Python sets and exploring some advanced techniques, you can take your coding skills to the next level and become a more proficient Python programmer. So why not start exploring the world of Python sets today and see how they can elevate your coding game?