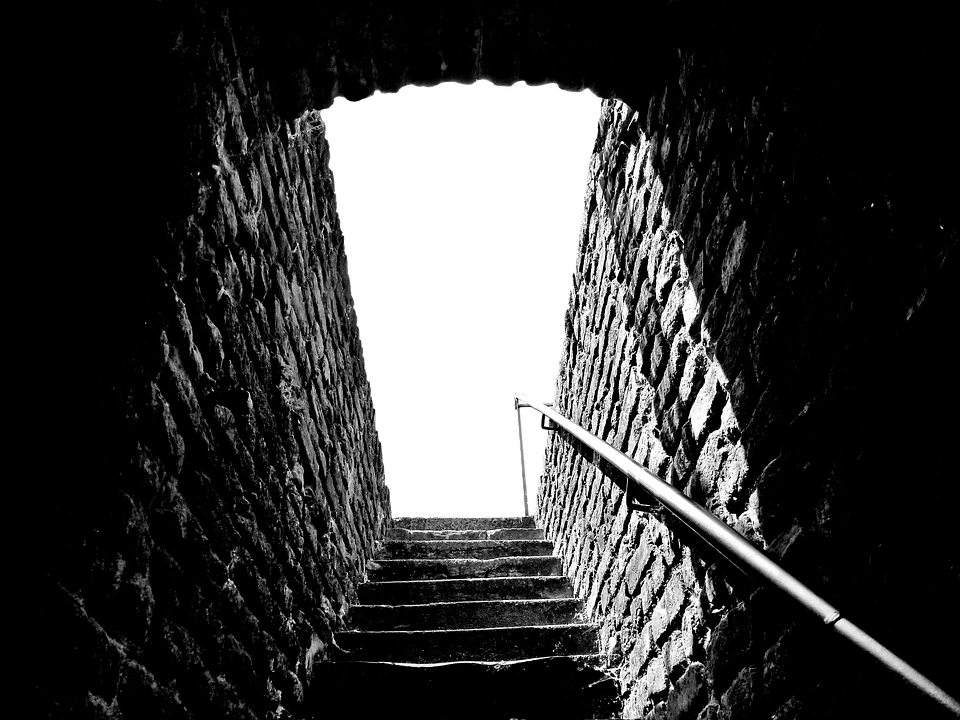
Concurrency is a powerful feature in programming languages that allows multiple tasks to execute simultaneously, leading to improved performance and efficiency. In the Go programming language, concurrency is a first-class citizen, making it easy for developers to write concurrent programs that take full advantage of modern multi-core processors.
One of the key features that makes Go a great language for concurrent programming is its built-in support for goroutines. Goroutines are lightweight threads of execution that can run concurrently with other goroutines within the same program. This makes it easy for developers to write concurrent code without having to worry about the low-level details of threading and synchronization.
To create a goroutine in Go, all you need to do is prefix a function call with the `go` keyword. For example, the following code snippet creates a new goroutine that prints “Hello, World!” concurrently with the main program:
“`go
func main() {
go fmt.Println(“Hello, World!”)
fmt.Println(“Main program”)
}
“`
In this example, the `fmt.Println(“Hello, World!”)` statement is executed in a separate goroutine, allowing it to run concurrently with the `fmt.Println(“Main program”)` statement in the main program. This allows both statements to be executed simultaneously, improving the overall performance of the program.
In addition to goroutines, Go also provides channels as a powerful mechanism for communication and synchronization between concurrent goroutines. Channels allow goroutines to send and receive data in a safe and synchronized manner, preventing race conditions and other concurrency-related bugs.
To create a channel in Go, you can use the `make` function with the `chan` keyword, like so:
“`go
ch := make(chan int)
“`
You can then use the channel to send and receive data between goroutines. For example, the following code snippet creates a channel, sends a value into the channel from one goroutine, and receives the value from another goroutine:
“`go
func main() {
ch := make(chan int)
go func() {
ch