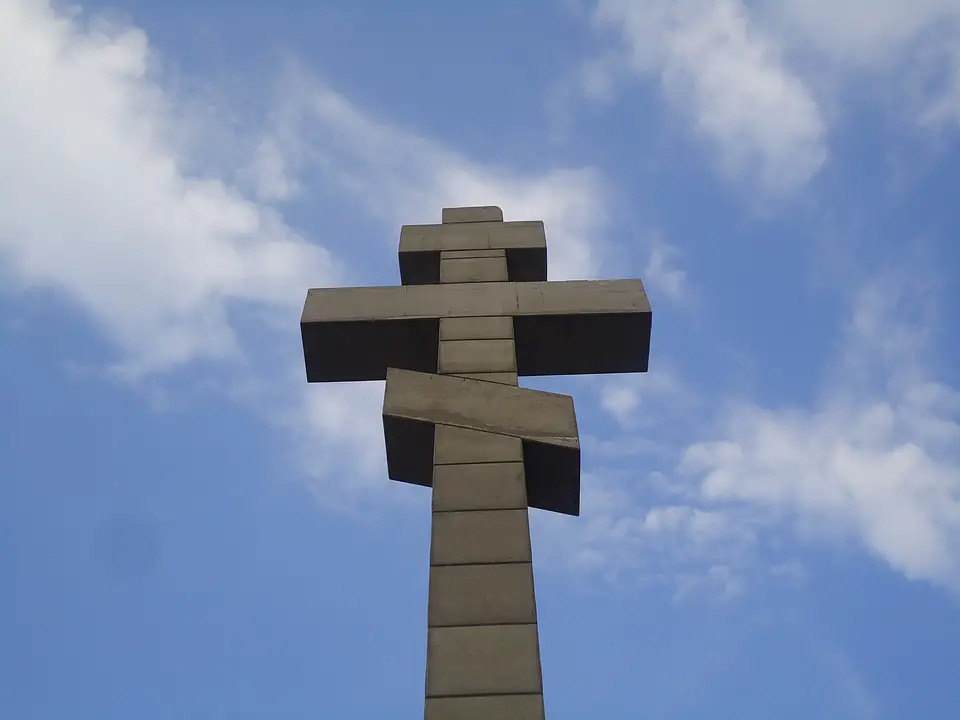
Python is a versatile and powerful programming language that is widely used for a variety of applications, from web development to data analysis. One of the key features of Python is its support for object-oriented programming, which allows developers to create reusable and modular code through the use of classes.
A class in Python is a blueprint for creating objects, which are instances of the class. Classes can contain attributes (variables) and methods (functions) that define the behavior of the objects created from the class. This makes classes a powerful tool for organizing and structuring code in a way that is easy to understand and maintain.
To create a class in Python, you use the `class` keyword followed by the name of the class. For example, you could create a simple class called `Person` like this:
“` python
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
print(f”Hello, my name is {self.name} and I am {self.age} years old.”)
“`
In this example, the `Person` class has two attributes, `name` and `age`, and one method, `greet`, which prints a greeting message using the attributes of the object. The `__init__` method is a special method called the constructor, which is used to initialize the attributes of the object when it is created.
To create an object from a class, you use the class name followed by parentheses, like this:
“` python
person1 = Person(“Alice”, 30)
person2 = Person(“Bob”, 25)
“`
You can then access the attributes and methods of the objects using dot notation, like this:
“` python
print(person1.name) # Output: Alice
person2.greet() # Output: Hello, my name is Bob and I am 25 years old.
“`
Classes can also inherit from other classes, allowing you to create hierarchies of classes with shared attributes and methods. For example, you could create a subclass of `Person` called `Employee` like this:
“` python
class Employee(Person):
def __init__(self, name, age, salary):
super().__init__(name, age)
self.salary = salary
def introduce(self):
print(f”My name is {self.name}, I am {self.age} years old, and my salary is {self.salary}.”)
“`
In this example, the `Employee` class inherits from the `Person` class, so it has access to the attributes and methods of the `Person` class. The `__init__` method of the `Employee` class calls the `__init__` method of the `Person` class using the `super()` function, and then initializes the `salary` attribute.
By exploring the ins and outs of Python classes, you can take advantage of the power and flexibility of object-oriented programming to create clear, organized, and maintainable code. Classes are a fundamental building block of Python programming, and mastering them will enable you to create more complex and sophisticated applications.