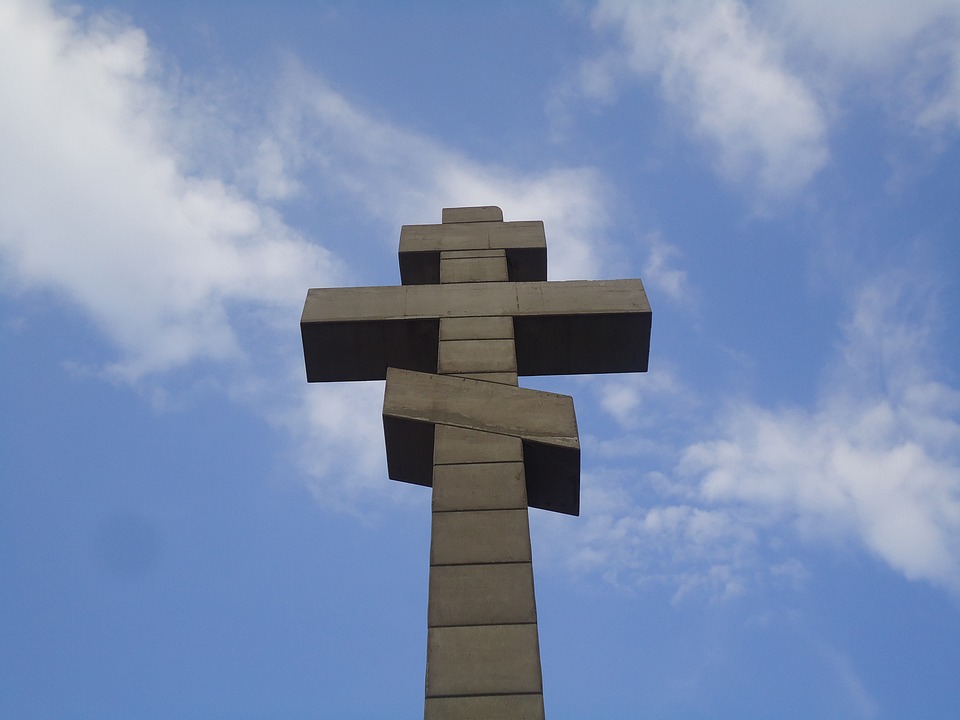
Python lists are a powerful data structure that allow for the storage and manipulation of multiple items. They are commonly used in a wide range of applications, from simple data storage to complex algorithmic computations. While basic list operations such as appending, indexing, and slicing are well-known, there are several advanced techniques that can further enhance the functionality and flexibility of Python lists.
1. List Comprehensions:
List comprehensions provide a concise way to create new lists based on existing ones. They allow for the inclusion of loops and conditional statements within a single line of code. For example, to create a new list with only the even numbers from an existing list, you can use a list comprehension like this:
“` python
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_numbers = [x for x in numbers if x % 2 == 0]
“`
This will result in `even_numbers` containing `[2, 4, 6, 8, 10]`. List comprehensions are not only concise, but also efficient, as they are implemented in C and can often outperform equivalent for loops.
2. Sorting Lists:
Python provides a built-in `sort()` function that can be used to sort lists in ascending order. However, sometimes we may need to sort lists in a custom order or based on specific criteria. In such cases, the `sorted()` function with a key parameter can be used. The key parameter allows you to specify a function that returns a value used for sorting. Here’s an example:
“` python
fruits = [‘apple’, ‘banana’, ‘cherry’, ‘date’]
sorted_fruits = sorted(fruits, key=len)
“`
The `key` parameter in this case is `len`, which specifies that the sorting should be based on the length of each element. The resulting `sorted_fruits` will be `[‘date’, ‘apple’, ‘banana’, ‘cherry’]`, as the elements are sorted in ascending order of their length.
3. Removing Duplicates:
Sometimes, we may need to remove duplicate elements from a list. Python makes this task simple by providing the `set()` function, which converts a list into a set, automatically removing any duplicates. However, this operation does not preserve the original order of the elements. To maintain the order while removing duplicates, we can use a combination of a list comprehension and a set. Here’s how it can be done:
“` python
numbers = [1, 2, 3, 4, 3, 2, 1]
unique_numbers = list(set(numbers))
“`
The resulting `unique_numbers` list will be `[1, 2, 3, 4]`, with the duplicate elements removed while preserving the original order.
4. Reversing Lists:
Python provides the `reverse()` method to reverse the order of elements in a list. However, this method modifies the original list in place. If you want to reverse a list without modifying the original, you can use slicing. Here’s an example:
“` python
numbers = [1, 2, 3, 4, 5]
reversed_numbers = numbers[::-1]
“`
The resulting `reversed_numbers` list will be `[5, 4, 3, 2, 1]`, with the order of elements reversed while keeping the original list intact.
These are just a few examples of the advanced techniques available for manipulating Python lists. By exploring and utilizing these techniques, you can enhance your coding efficiency and create more powerful and flexible Python programs.