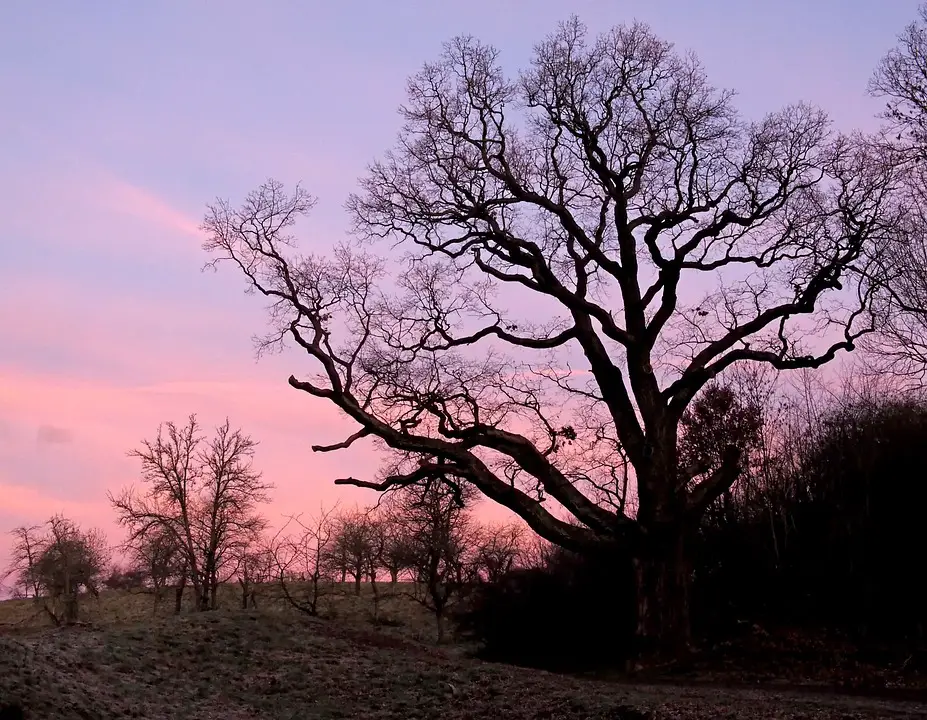
Python is a versatile and powerful programming language that is widely used in various fields, including data science, machine learning, web development, and more. One of the key features of Python is its ability to work with lists, which are ordered collections of items. In this article, we will explore some advanced list manipulation techniques that can help you enhance your Python skills.
1. List comprehension: List comprehension is a concise way to create lists in Python. It allows you to create a new list by applying an expression to each item in an existing list. For example, you can use list comprehension to create a list of squares of numbers from 1 to 10:
“` python
squares = [x**2 for x in range(1, 11)]
print(squares)
“`
2. Filtering lists: You can use list comprehension to filter elements from a list based on a condition. For example, you can create a list of even numbers from a list of numbers:
“` python
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_numbers = [x for x in numbers if x % 2 == 0]
print(even_numbers)
“`
3. Mapping lists: You can also use list comprehension to apply a function to each item in a list. For example, you can convert a list of strings to uppercase:
“` python
names = [‘alice’, ‘bob’, ‘charlie’]
uppercase_names = [name.upper() for name in names]
print(uppercase_names)
“`
4. Nested lists: Python allows you to create nested lists, which are lists that contain other lists. You can use nested lists to represent more complex data structures. For example, you can create a matrix using nested lists:
“` python
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
print(matrix)
“`
5. List slicing: List slicing allows you to access a subset of elements from a list. You can specify a start index, an end index, and a step size. For example, you can extract every other element from a list:
“` python
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
every_other_number = numbers[::2]
print(every_other_number)
“`
By mastering these advanced list manipulation techniques, you can write more concise and efficient code in Python. These techniques will not only help you improve your programming skills but also make you a more effective and productive Python developer. So, start practicing these techniques and take your Python skills to the next level!