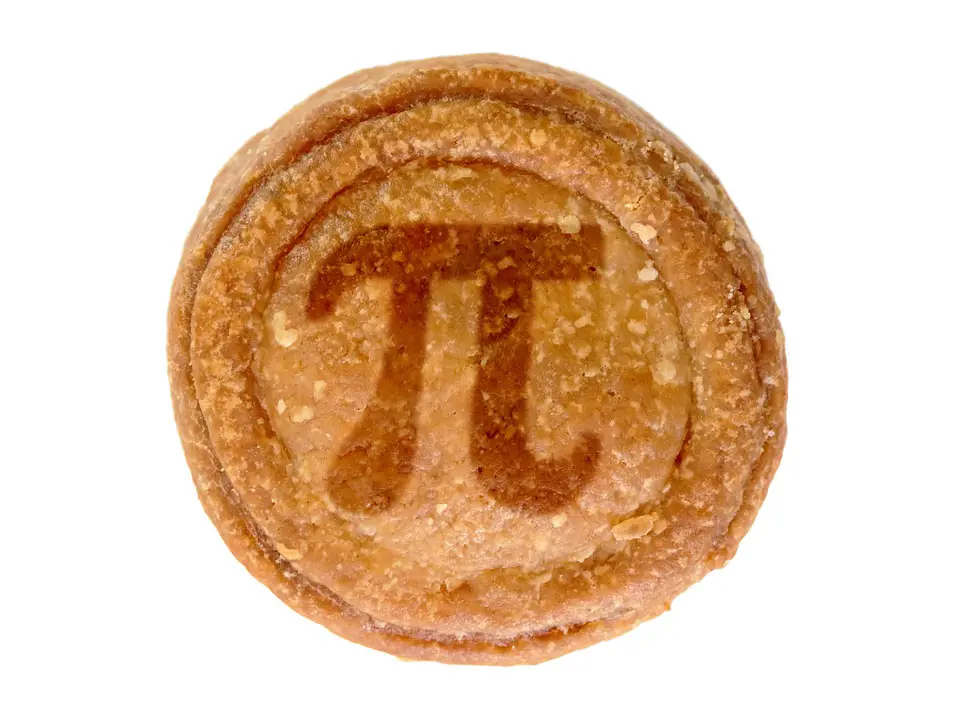
Python is a versatile and powerful programming language that is widely used in the software development industry. One of the key features of Python is its ability to manipulate lists efficiently. Lists are a fundamental data structure in Python, and mastering list manipulation techniques can greatly enhance your Python programming skills.
Here are some useful list manipulation techniques that you can use to improve your Python skills:
1. List comprehension: List comprehension is a concise way to create lists in Python. It allows you to generate new lists by applying an expression to each element of an existing list. For example, the following code snippet creates a new list containing the squares of numbers from 1 to 5:
“` python
squares = [x**2 for x in range(1, 6)]
print(squares)
“`
2. Filtering lists: You can use list comprehension to filter elements from a list based on a condition. For example, the following code snippet creates a new list containing only the even numbers from a list of integers:
“` python
numbers = [1, 2, 3, 4, 5, 6]
even_numbers = [x for x in numbers if x % 2 == 0]
print(even_numbers)
“`
3. Sorting lists: Python provides a built-in `sort()` method that allows you to sort a list in ascending order. You can also use the `sorted()` function to create a new sorted list without modifying the original list. For example, the following code snippet sorts a list of strings alphabetically:
“` python
fruits = [‘apple’, ‘banana’, ‘orange’, ‘kiwi’]
sorted_fruits = sorted(fruits)
print(sorted_fruits)
“`
4. Reversing lists: Python provides a built-in `reverse()` method that allows you to reverse the order of elements in a list. For example, the following code snippet reverses a list of integers:
“` python
numbers = [1, 2, 3, 4, 5]
numbers.reverse()
print(numbers)
“`
5. Combining lists: You can use the `extend()` method to append elements from one list to another list. For example, the following code snippet combines two lists of integers:
“` python
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list1.extend(list2)
print(list1)
“`
By mastering these list manipulation techniques, you can become more proficient in Python programming and improve your ability to work with data in your projects. Practice using these techniques in your code to gain a deeper understanding of how lists work in Python and enhance your programming skills.