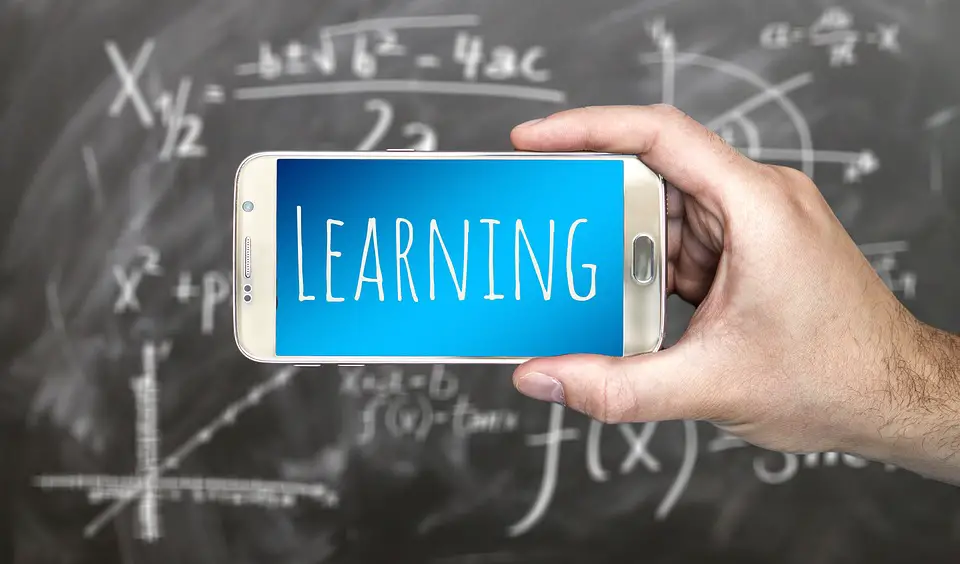
Python’s map function is a powerful tool that allows developers to apply a function to each element in an iterable, such as a list or tuple. While map is commonly used for simple operations, such as multiplying each element in a list by a constant, experienced developers can take advantage of more advanced techniques to fully harness the power of this function.
One of the key benefits of using map is that it can significantly reduce the amount of code needed to perform certain operations. For example, instead of writing a loop to iterate over a list and apply a function to each element, you can use map to achieve the same result in a single line of code. This can make your code more concise and easier to read, as well as potentially improving performance.
One advanced technique for using map is to combine it with lambda functions. Lambda functions are anonymous functions that can be defined inline, allowing you to create simple functions on the fly. By using lambda functions with map, you can quickly and easily apply custom operations to each element in an iterable. For example, you could use a lambda function to square each element in a list:
“`
numbers = [1, 2, 3, 4, 5]
squared_numbers = list(map(lambda x: x**2, numbers))
“`
Another advanced technique is to use map with multiple iterables. While map typically only takes a single iterable as input, you can use the zip function to combine multiple iterables into a single iterable of tuples. This allows you to apply a function that takes multiple arguments to corresponding elements in each iterable. For example, you could use map with zip to add corresponding elements from two lists:
“`
numbers1 = [1, 2, 3, 4, 5]
numbers2 = [6, 7, 8, 9, 10]
sums = list(map(lambda x, y: x + y, numbers1, numbers2))
“`
By diving deeper into Python map and exploring these advanced techniques, experienced developers can take their coding skills to the next level. Whether you’re looking to streamline your code, apply custom operations to iterables, or work with multiple iterables simultaneously, map is a versatile tool that can help you achieve your goals. So next time you find yourself writing a loop to iterate over a list, consider using map instead and see how it can simplify and enhance your code.