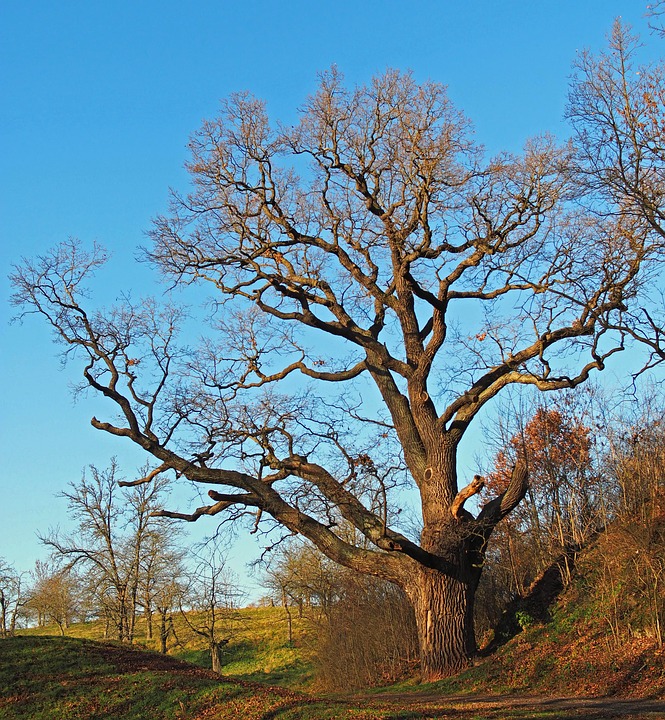
Python sets are a powerful and versatile data structure that is often underutilized by beginner programmers. Understanding how sets work and how to effectively use them can greatly improve your coding skills and make your programs more efficient. In this article, we will demystify Python sets and provide a beginner’s guide to understanding and utilizing them.
What is a set in Python?
A set in Python is an unordered collection of unique elements. This means that a set can only contain unique values, and duplicate elements are automatically removed. Sets are mutable, meaning that you can add or remove elements from a set after it has been created.
Sets are defined using curly braces {} and can contain any data type, including numbers, strings, and even other sets. For example, a set containing the numbers 1, 2, and 3 would be defined as follows:
my_set = {1, 2, 3}
It’s important to note that sets do not support indexing, meaning that you cannot access individual elements in a set using an index. Instead, you can use methods like add() and remove() to modify the contents of a set.
Why use sets in Python?
Sets are incredibly useful in Python for a variety of reasons. One of the main advantages of using sets is their ability to efficiently check for membership of an element. Because sets only contain unique values, you can quickly determine whether a specific element is present in a set without having to iterate through the entire collection.
Sets are also useful for performing set operations, such as union, intersection, and difference. These operations allow you to combine or compare multiple sets to create new sets with different elements. For example, you can use the union() method to combine two sets into a single set containing all unique elements from both sets.
How to work with sets in Python
Now that you understand the basics of sets in Python, let’s explore some common operations and methods that you can use to manipulate sets in your programs.
Creating a set: To create a set in Python, you can simply use curly braces {} and separate the elements with commas. For example:
my_set = {1, 2, 3}
Adding elements to a set: You can add elements to a set using the add() method. For example:
my_set.add(4)
Removing elements from a set: You can remove elements from a set using the remove() method. For example:
my_set.remove(2)
Checking for membership: You can check if a specific element is present in a set using the in keyword. For example:
if 1 in my_set:
print(“1 is in the set”)
Set operations: You can perform set operations like union, intersection, and difference using built-in methods. For example:
set1 = {1, 2, 3}
set2 = {3, 4, 5}
union_set = set1.union(set2)
intersection_set = set1.intersection(set2)
difference_set = set1.difference(set2)
In conclusion, Python sets are a powerful and versatile data structure that can greatly improve the efficiency and readability of your code. By understanding how sets work and how to effectively use them, you can take your programming skills to the next level and create more robust and efficient programs. I hope this beginner’s guide has helped demystify Python sets and given you the knowledge you need to start using them in your own projects.