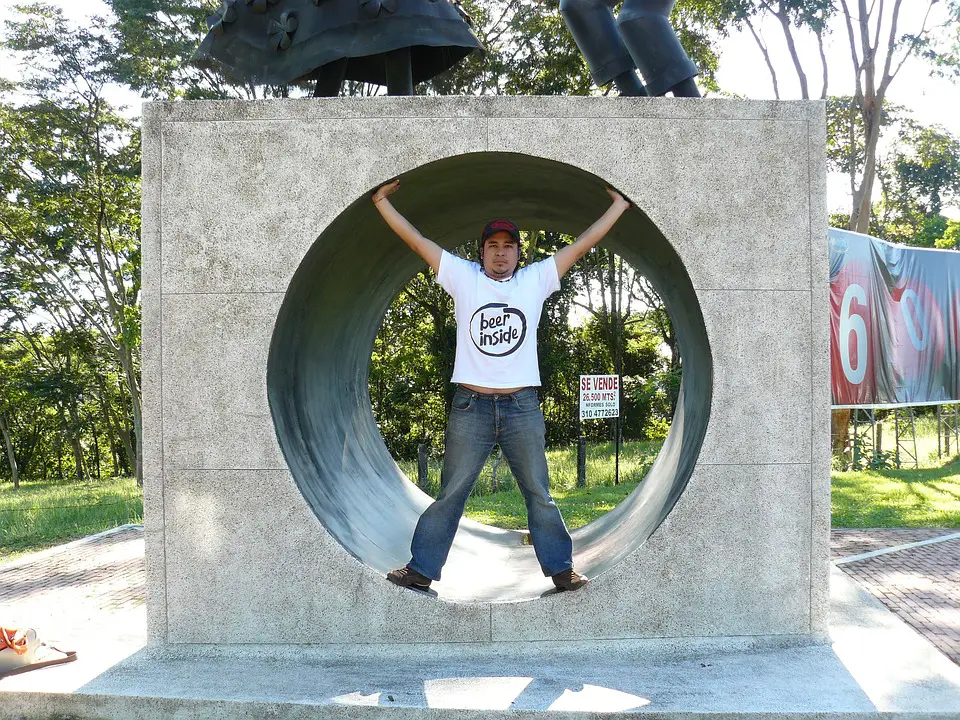
Python is a popular programming language known for its simplicity and readability. One of the most commonly used data structures in Python is the list. Lists are versatile containers that can hold a collection of items, such as integers, strings, or even other lists. In this article, we will demystify Python lists and cover everything you need to know about them.
Creating a List
To create a list in Python, you can simply enclose a comma-separated sequence of items inside square brackets. For example, to create a list of integers, you can do the following:
“` python
my_list = [1, 2, 3, 4, 5]
“`
You can also create a list of strings:
“` python
my_list = [‘apple’, ‘banana’, ‘cherry’]
“`
Accessing Elements
You can access elements in a list by their index. In Python, indexing starts at 0, so the first element in a list has an index of 0, the second element has an index of 1, and so on. To access a specific element in a list, you can use square brackets with the index of the element you want to retrieve. For example:
“` python
my_list = [1, 2, 3, 4, 5]
print(my_list[0]) # Output: 1
print(my_list[2]) # Output: 3
“`
You can also use negative indexing to access elements from the end of the list. For example, `my_list[-1]` will give you the last element in the list.
Modifying Lists
Lists in Python are mutable, which means you can modify their elements. You can change the value of an element by assigning a new value to it. For example:
“` python
my_list = [1, 2, 3, 4, 5]
my_list[2] = 10
print(my_list) # Output: [1, 2, 10, 4, 5]
“`
You can also add new elements to a list using the `append()` method. For example:
“` python
my_list = [1, 2, 3, 4, 5]
my_list.append(6)
print(my_list) # Output: [1, 2, 3, 4, 5, 6]
“`
List Comprehensions
List comprehensions are a concise way to create lists in Python. They allow you to generate a new list by applying an expression to each item in an existing list. For example, you can create a list of squared numbers using a list comprehension:
“` python
squared_numbers = [x**2 for x in range(1, 6)]
print(squared_numbers) # Output: [1, 4, 9, 16, 25]
“`
List Methods
Python lists come with several built-in methods that allow you to manipulate and work with lists efficiently. Some common list methods include `sort()`, `reverse()`, `pop()`, `remove()`, and `count()`. You can find a complete list of list methods in the Python documentation.
In conclusion, Python lists are versatile and powerful data structures that can be used to store and manipulate collections of items. By understanding how to create, access, modify, and work with lists in Python, you can write more efficient and elegant code. We hope this article has demystified Python lists and provided you with everything you need to know about them. Happy coding!