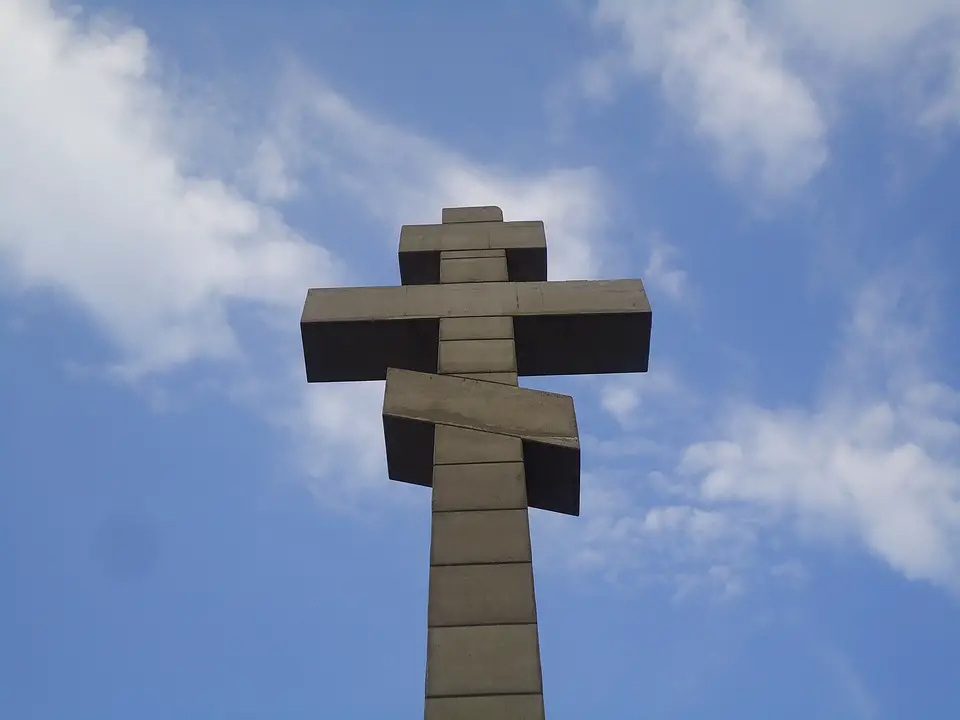
Python is a versatile and powerful programming language that is widely used in various fields such as web development, data analysis, and artificial intelligence. One of the key features of Python is its support for object-oriented programming, which allows developers to create reusable and organized code by defining classes and objects.
If you are new to Python or object-oriented programming, understanding classes can be a daunting task. However, with a clear understanding of the fundamentals, you can demystify Python classes and harness their power in your programming projects.
What is a Class?
In Python, a class is a blueprint for creating objects. It defines the structure and behavior of objects by specifying attributes (variables) and methods (functions) that belong to the class. Think of a class as a template that can be used to create multiple instances of objects with similar properties.
Defining a Class in Python
To define a class in Python, you use the class keyword followed by the class name. Inside the class definition, you can define attributes and methods that belong to the class. Here is an example of a simple class definition in Python:
“` python
class Car:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
def display_info(self):
print(f”{self.year} {self.make} {self.model}”)
“`
In this example, we defined a Car class with three attributes (make, model, and year) and a method (display_info) that prints the information about the car.
Creating Objects from a Class
Once you have defined a class, you can create objects (instances) of that class by calling the class name followed by parentheses. Here is how you can create an instance of the Car class from the previous example:
“` python
my_car = Car(“Tesla”, “Model 3”, 2021)
“`
Accessing Attributes and Calling Methods
You can access the attributes of an object using dot notation. For example, to access the make attribute of the my_car object, you can use the following code:
“` python
print(my_car.make)
“`
Similarly, you can call methods of an object using dot notation. To display information about the car, you can call the display_info method as follows:
“` python
my_car.display_info()
“`
Understanding the __init__ Method
In the previous example, we defined a special method called __init__ (short for initialization) inside the class. This method is known as a constructor and is called automatically when an object is created. It is used to initialize the attributes of the object. The self parameter refers to the object itself and is used to access its attributes and methods.
In conclusion, understanding the fundamentals of Python classes is essential for mastering object-oriented programming in Python. By defining classes, creating objects, and accessing attributes and methods, you can organize your code effectively and create reusable components for your projects. With practice and experimentation, you can harness the power of Python classes to build complex and efficient software solutions.