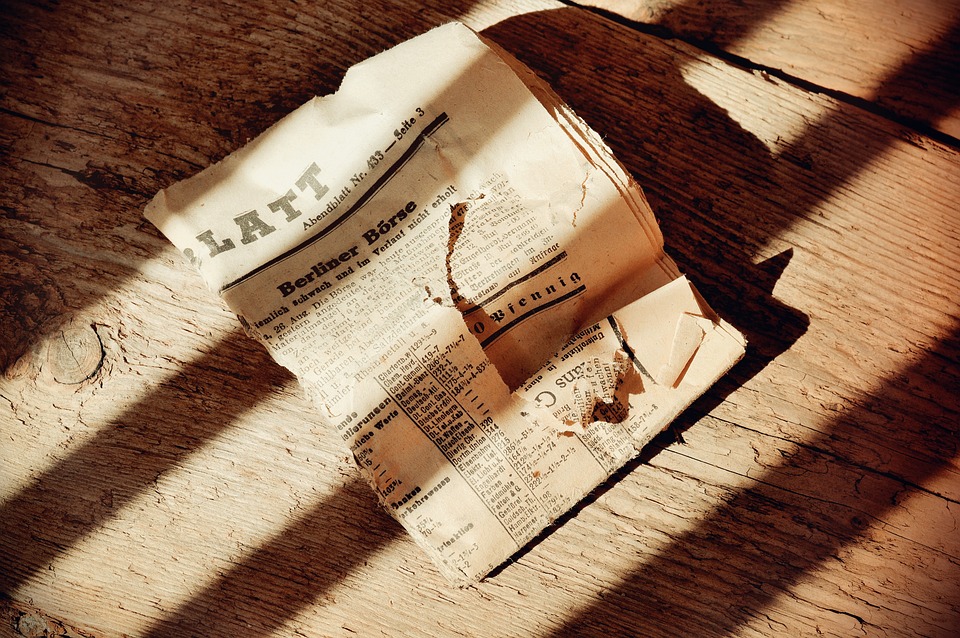
JavaScript’s reduce method is a powerful and versatile function that can be used to perform a wide range of operations on arrays. Despite its usefulness, many beginners find the reduce method confusing and intimidating. In this article, we will demystify the reduce method and provide a beginner’s guide on how to use it effectively.
The reduce method is a higher-order function that takes an array and a callback function as its arguments. The callback function in turn takes two parameters: an accumulator and the current value in the array being processed. The reduce method then iterates over the array, applying the callback function to each element and updating the accumulator along the way.
One of the most common use cases for the reduce method is to sum up all the values in an array. For example, consider the following array:
“`javascript
const numbers = [1, 2, 3, 4, 5];
“`
To calculate the sum of all the numbers in the array, we can use the reduce method like so:
“`javascript
const sum = numbers.reduce((acc, curr) => acc + curr, 0);
“`
In this example, the accumulator (acc) starts at 0 and is updated with the current value (curr) in each iteration. The result is the sum of all the numbers in the array, which is stored in the variable sum.
Another common use case for the reduce method is to find the maximum value in an array. For example, consider the following array:
“`javascript
const numbers = [10, 5, 20, 15, 30];
“`
To find the maximum value in the array, we can use the reduce method like so:
“`javascript
const max = numbers.reduce((acc, curr) => Math.max(acc, curr), -Infinity);
“`
In this example, we use the Math.max function within the callback function to compare the current value with the accumulator and return the larger of the two. The result is the maximum value in the array, which is stored in the variable max.
The reduce method can also be used to transform an array into a different data structure, such as an object. For example, consider the following array of objects:
“`javascript
const products = [
{ id: 1, name: ‘Product 1’ },
{ id: 2, name: ‘Product 2’ },
{ id: 3, name: ‘Product 3’ }
];
“`
To transform this array into an object where the keys are the product IDs and the values are the product names, we can use the reduce method like so:
“`javascript
const productMap = products.reduce((acc, curr) => {
acc[curr.id] = curr.name;
return acc;
}, {});
“`
In this example, we start with an empty object as the accumulator and add a key-value pair for each product in the array. The result is an object where the keys are the product IDs and the values are the product names, which is stored in the variable productMap.
In conclusion, the reduce method is a powerful tool in JavaScript that allows you to perform a wide range of operations on arrays. By understanding how the reduce method works and practicing with different use cases, you can become more comfortable and proficient in using this function in your code. With this beginner’s guide, you can now confidently demystify the reduce method and leverage its capabilities in your JavaScript projects.