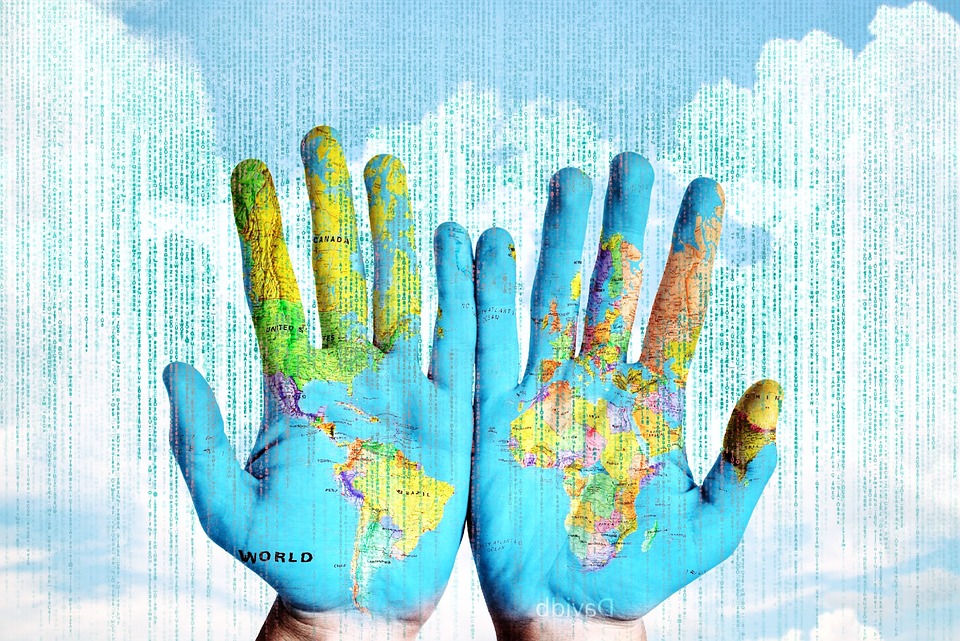
JavaScript is a versatile and powerful programming language that is commonly used in web development. One of the many useful methods in JavaScript is the split() method. In this article, we will demystify the split() method and provide a comprehensive guide on how to use it effectively in your code.
The split() method in JavaScript is used to split a string into an array of substrings based on a specified delimiter. The syntax for the split() method is as follows:
string.split(separator, limit)
The first parameter, separator, is a string that specifies where to split the original string. This can be a single character, multiple characters, or a regular expression. If the separator is omitted, the entire string is split into individual characters.
The second parameter, limit, is an optional parameter that specifies the maximum number of splits to be performed. If the limit is provided, the split() method will stop splitting the string after the specified number of splits have been made.
Here’s an example of how to use the split() method:
“`
let str = “Hello,World,JavaScript”;
let arr = str.split(“,”);
console.log(arr);
“`
In this example, the string “Hello,World,JavaScript” is split into an array of substrings based on the comma delimiter. The resulting array would be [“Hello”, “World”, “JavaScript”].
You can also use regular expressions as the separator in the split() method. For example:
“`
let str = “Hello World JavaScript”;
let arr = str.split(/\s/);
console.log(arr);
“`
In this example, the string “Hello World JavaScript” is split into an array of substrings based on whitespace characters. The resulting array would be [“Hello”, “World”, “JavaScript”].
It’s important to note that the split() method does not change the original string. Instead, it returns a new array of substrings. If you want to modify the original string, you can use the join() method to concatenate the array elements back into a string.
The split() method is a powerful tool in JavaScript that can be used in a variety of scenarios. It can be used to parse data from a CSV file, extract information from a URL, or split a string into individual words for further processing.
In conclusion, the split() method in JavaScript is a versatile and useful tool for splitting strings into arrays of substrings. By understanding how to use the split() method effectively, you can enhance your coding skills and create more dynamic and interactive web applications.