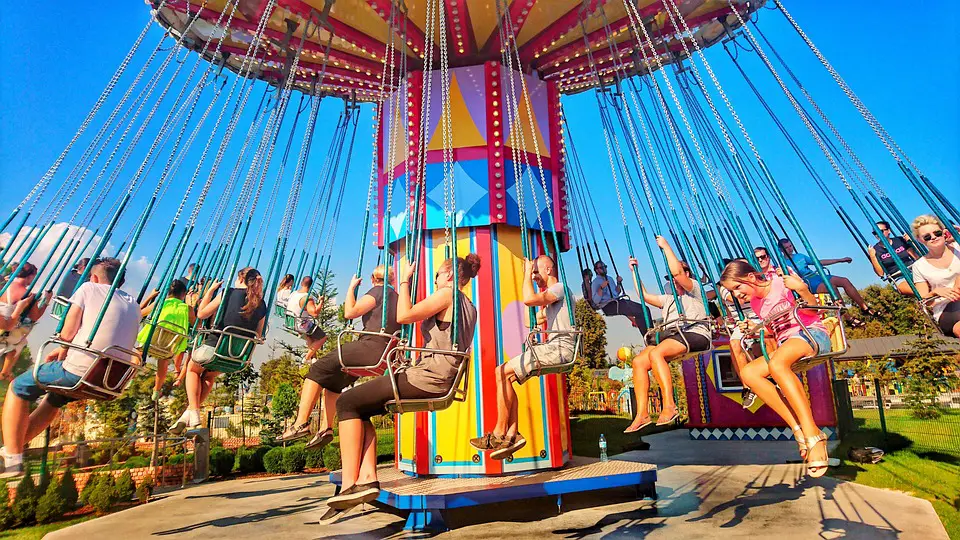
Go, also known as Golang, is a powerful programming language that is gaining popularity among developers for its simplicity, speed, and efficiency. With its built-in support for concurrency and its fast compilation times, Go is an excellent choice for building web applications that need to handle a large number of concurrent requests.
In this article, we will walk through step-by-step examples and tutorials for creating web applications with Go. Whether you are new to Go or have some experience with the language, these examples will help you get started with building web applications using Go.
Setting up your Go environment
Before you start building web applications with Go, you need to set up your development environment. The first step is to install Go on your machine. You can download the latest version of Go from the official Go website and follow the installation instructions for your operating system.
Once you have installed Go, you can create a new directory for your web application and set up your Go workspace. You can do this by setting the GOPATH environment variable to point to the directory where you want to store your Go projects. For example, you can set GOPATH to ~/go in your .bashrc or .zshrc file.
Creating a simple web server
Now that you have set up your Go environment, you can start building your web application. The first step is to create a simple web server using the net/http package in Go. Here is an example of a basic web server in Go:
“`go
package main
import (
“fmt”
“net/http”
)
func handler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, “Hello, World!”)
}
func main() {
http.HandleFunc(“/”, handler)
http.ListenAndServe(“:8080”, nil)
}
“`
In this example, we define a handler function that writes “Hello, World!” to the response writer w. We then use the http.HandleFunc function to register the handler function with the root path “/” and start the web server using http.ListenAndServe on port 8080.
You can run this code by saving it to a file named main.go and running go run main.go in your terminal. You should see a message that the server is running on http://localhost:8080. You can open this URL in your web browser to see the “Hello, World!” message displayed.
Creating a dynamic web application
Now that you have created a simple web server in Go, you can start building more complex web applications. One common use case for web applications is to display dynamic content based on user input. Here is an example of a dynamic web application in Go that displays a personalized greeting based on the user’s name:
“`go
package main
import (
“fmt”
“net/http”
)
func handler(w http.ResponseWriter, r *http.Request) {
name := r.URL.Query().Get(“name”)
if name == “” {
name = “World”
}
fmt.Fprintf(w, “Hello, %s!”, name)
}
func main() {
http.HandleFunc(“/”, handler)
http.ListenAndServe(“:8080”, nil)
}
“`
In this example, we extract the value of the “name” query parameter from the request URL using r.URL.Query().Get(“name”). If the “name” parameter is not provided, we default to “World”. We then display a personalized greeting using fmt.Fprintf(w, “Hello, %s!”, name).
You can run this code in the same way as before and pass a name parameter in the URL to see a personalized greeting. For example, you can open http://localhost:8080/?name=John in your web browser to see the message “Hello, John!” displayed.
Conclusion
In this article, we have walked through step-by-step examples and tutorials for creating web applications with Go. By following these examples, you can learn how to set up your Go environment, create a simple web server, and build dynamic web applications using Go’s powerful features.
Whether you are new to Go or have some experience with the language, these examples will help you get started with building web applications with Go. With its simplicity, speed, and efficiency, Go is an excellent choice for developing web applications that need to handle a large number of concurrent requests. So why wait? Start building your web applications with Go today!