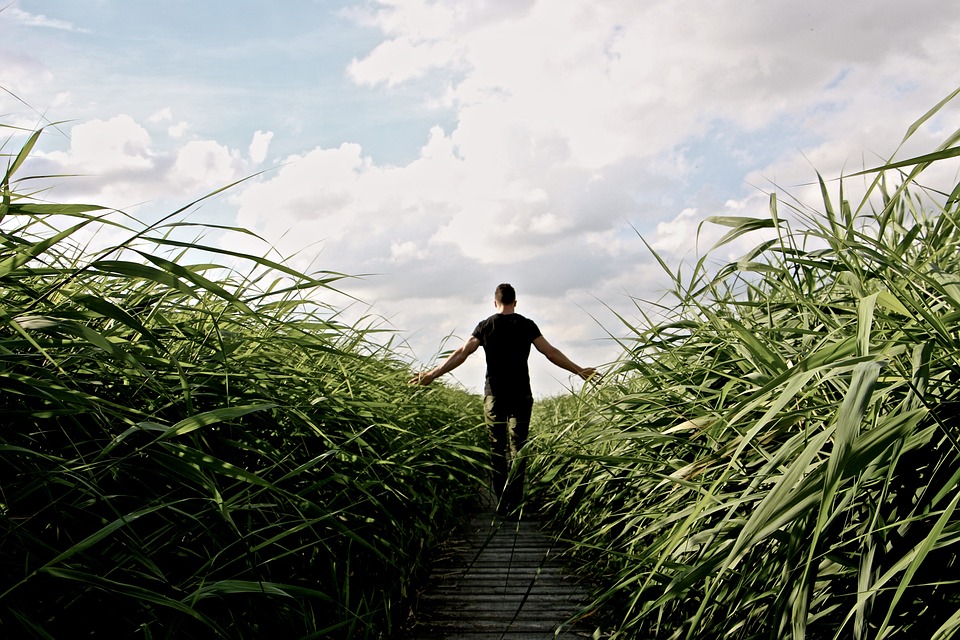
Microservices architecture has gained popularity in recent years as a way to build applications that are flexible, scalable, and easy to maintain. By breaking down a monolithic application into smaller, independent services that communicate with each other through APIs, developers can build complex applications that are easier to develop and deploy.
One popular language for building microservices is Go (or Golang). Go is a statically typed, compiled language that was developed by Google and is known for its simplicity, efficiency, and performance. In this article, we will explore some examples of building scalable microservices in Go and discuss some best practices for designing and implementing microservices in Go.
Example of building a simple microservice in Go:
Let’s start by building a simple microservice in Go that exposes an API endpoint to get a list of users. We will use the popular Gorilla Mux router for handling HTTP requests.
“`go
package main
import (
“encoding/json”
“log”
“net/http”
“github.com/gorilla/mux”
)
type User struct {
ID string `json:”id”`
Name string `json:”name”`
}
var users = []User{
{ID: “1”, Name: “Alice”},
{ID: “2”, Name: “Bob”},
}
func getUsers(w http.ResponseWriter, r *http.Request) {
w.Header().Set(“Content-Type”, “application/json”)
json.NewEncoder(w).Encode(users)
}
func main() {
r := mux.NewRouter()
r.HandleFunc(“/users”, getUsers).Methods(“GET”)
log.Fatal(http.ListenAndServe(“:8080”, r))
}
“`
In this example, we define a User struct to represent a user and a slice of users. We then create a handler function getUsers that returns the list of users in JSON format. We use the Gorilla Mux router to define a route for the /users endpoint that calls the getUsers function.
Best practices for building microservices in Go:
1. Keep microservices small and focused: Each microservice should have a single responsibility and do it well. This makes it easier to understand, test, and maintain the codebase.
2. Use interfaces for dependencies: By defining interfaces for dependencies, you can easily swap out implementations and mock dependencies for testing.
3. Use context.Context for request-scoped values: Use the context package in Go to pass request-scoped values, such as request ID or user authentication, through your microservices.
4. Use a logging framework: Use a logging framework like Logrus or Zap to log errors, warnings, and other information in a structured way.
5. Use a monitoring and alerting system: Use tools like Prometheus and Grafana to monitor the performance and health of your microservices and set up alerts for critical issues.
By following these best practices and examples, you can build scalable microservices in Go that are easy to develop, maintain, and deploy. Go’s simplicity, efficiency, and performance make it an ideal language for building microservices that can handle high traffic and complex applications.