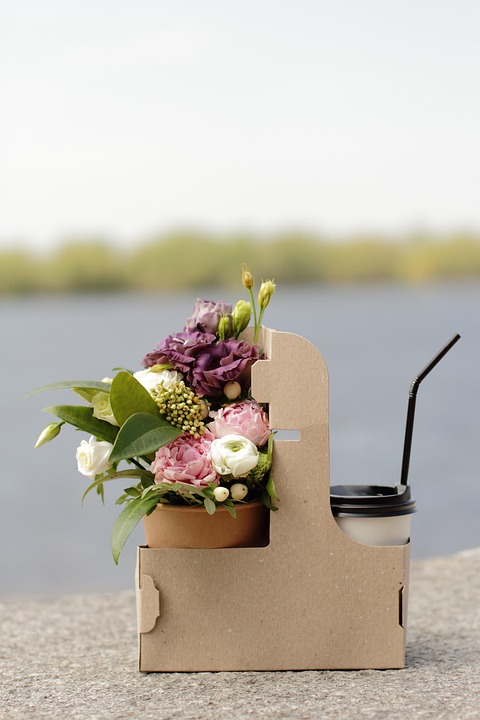
Microservices architecture has gained popularity in recent years as a way to build scalable and easily maintainable applications. By breaking down a monolithic application into smaller, independent services, development teams can work on different parts of the application simultaneously, leading to faster development cycles and easier updates.
One popular language for building microservices is Go, also known as Golang. Go is a statically typed, compiled language that is known for its speed, simplicity, and efficiency. In this article, we will explore how to build microservices in Go, including code examples and implementation tips.
1. Setting up a basic microservice
To get started with building a microservice in Go, you will need to set up a basic HTTP server. Below is an example of how you can create a simple microservice that returns a “Hello, World!” message when accessed.
“`go
package main
import (
“fmt”
“net/http”
)
func handler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, “Hello, World!”)
}
func main() {
http.HandleFunc(“/”, handler)
http.ListenAndServe(“:8080”, nil)
}
“`
In this example, we define a handler function that writes the “Hello, World!” message to the ResponseWriter. We then use the http.HandleFunc method to associate the handler function with the “/” route and start the HTTP server with the http.ListenAndServe method.
2. Implementing a RESTful API
To build a more complex microservice that interacts with a database and exposes a RESTful API, you can use a library like Gorilla Mux for routing. Here is an example of how you can create a simple CRUD API using Gorilla Mux.
“`go
package main
import (
“fmt”
“log”
“net/http”
“github.com/gorilla/mux”
)
func GetHandler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, “Get request”)
}
func PostHandler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, “Post request”)
}
func PutHandler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, “Put request”)
}
func DeleteHandler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, “Delete request”)
}
func main() {
r := mux.NewRouter()
r.HandleFunc(“/get”, GetHandler).Methods(“GET”)
r.HandleFunc(“/post”, PostHandler).Methods(“POST”)
r.HandleFunc(“/put”, PutHandler).Methods(“PUT”)
r.HandleFunc(“/delete”, DeleteHandler).Methods(“DELETE”)
log.Fatal(http.ListenAndServe(“:8080”, r))
}
“`
In this example, we use Gorilla Mux to define different routes for handling GET, POST, PUT, and DELETE requests. Each route is associated with a specific handler function that processes the request and returns a response.
3. Handling errors and logging
When building microservices, it is important to handle errors properly and log relevant information for debugging purposes. You can use the standard log package in Go to log errors and other messages.
“`go
package main
import (
“log”
“net/http”
)
func handler(w http.ResponseWriter, r *http.Request) {
_, err := w.Write([]byte(“Hello, World!”))
if err != nil {
log.Printf(“Error writing response: %v”, err)
http.Error(w, “Internal server error”, http.StatusInternalServerError)
}
}
func main() {
http.HandleFunc(“/”, handler)
http.ListenAndServe(“:8080”, nil)
}
“`
In this example, we use the log package to log an error message if there is an issue writing the response. We also use the http.Error method to return a 500 Internal Server Error response in case of an error.
Building microservices in Go can be a rewarding experience, thanks to the language’s simplicity and efficiency. By following these code examples and implementation tips, you can create robust and scalable microservices that meet the needs of your application. Remember to test your microservices thoroughly and monitor their performance to ensure they are running smoothly in production.