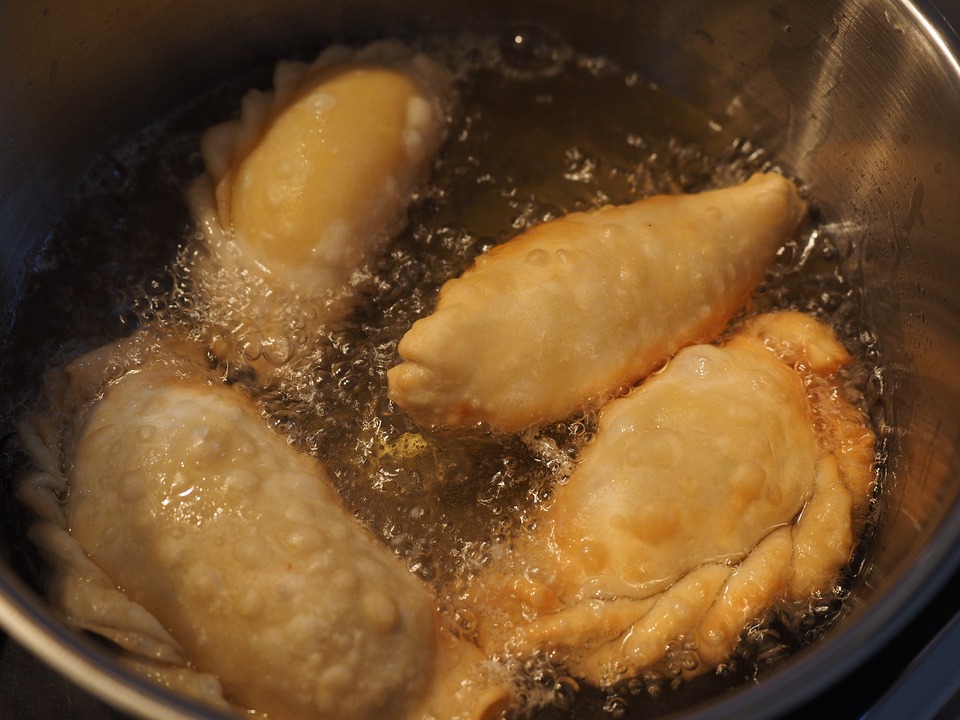
Deep learning is a powerful subset of artificial intelligence that has revolutionized many industries, from healthcare to finance to transportation. However, for many people, the concept of deep learning can seem overwhelming and complex. In this article, we will break down the complex concepts of deep learning and show you how to implement it using Python, a popular programming language for data science and machine learning.
At its core, deep learning is a type of machine learning that uses neural networks to learn from data. Neural networks are mathematical models inspired by the structure and function of the human brain. They consist of layers of interconnected nodes, or neurons, that process and transform input data to make predictions or classifications.
One of the key advantages of deep learning is its ability to automatically learn representations of data, without the need for manual feature engineering. This means that deep learning models can learn complex patterns and relationships in data that may be difficult for humans to specify explicitly.
To implement deep learning in Python, we can use libraries such as TensorFlow, Keras, and PyTorch. These libraries provide high-level abstractions and tools for building, training, and deploying deep learning models.
Let’s walk through a simple example to demonstrate how to build a basic deep learning model in Python. We will use the popular MNIST dataset, which consists of handwritten digits from 0 to 9.
First, we need to import the necessary libraries:
“` python
import tensorflow as tf
from tensorflow.keras import layers, models
“`
Next, we will load the MNIST dataset:
“` python
mnist = tf.keras.datasets.mnist
(x_train, y_train), (x_test, y_test) = mnist.load_data()
“`
Now, we will preprocess the data by normalizing and reshaping it:
“` python
x_train, x_test = x_train / 255.0, x_test / 255.0
x_train = x_train.reshape(x_train.shape[0], 28, 28, 1)
x_test = x_test.reshape(x_test.shape[0], 28, 28, 1)
“`
Next, we will define our deep learning model using a convolutional neural network (CNN) architecture:
“` python
model = models.Sequential([
layers.Conv2D(32, (3, 3), activation=’relu’, input_shape=(28, 28, 1)),
layers.MaxPooling2D((2, 2)),
layers.Conv2D(64, (3, 3), activation=’relu’),
layers.MaxPooling2D((2, 2)),
layers.Flatten(),
layers.Dense(128, activation=’relu’),
layers.Dense(10, activation=’softmax’)
])
“`
Finally, we will compile and train the model on the MNIST dataset:
“` python
model.compile(optimizer=’adam’,
loss=’sparse_categorical_crossentropy’,
metrics=[‘accuracy’])
model.fit(x_train, y_train, epochs=5)
“`
By following these simple steps, we have built a deep learning model in Python that can accurately classify handwritten digits. This example demonstrates how powerful and accessible deep learning can be with the right tools and knowledge.
In conclusion, deep learning may seem like a complex and intimidating concept, but with the right approach and tools, anyone can learn how to implement it. By breaking down the complex concepts of deep learning and providing practical examples in Python, we hope to empower more people to explore and harness the potential of this transformative technology.