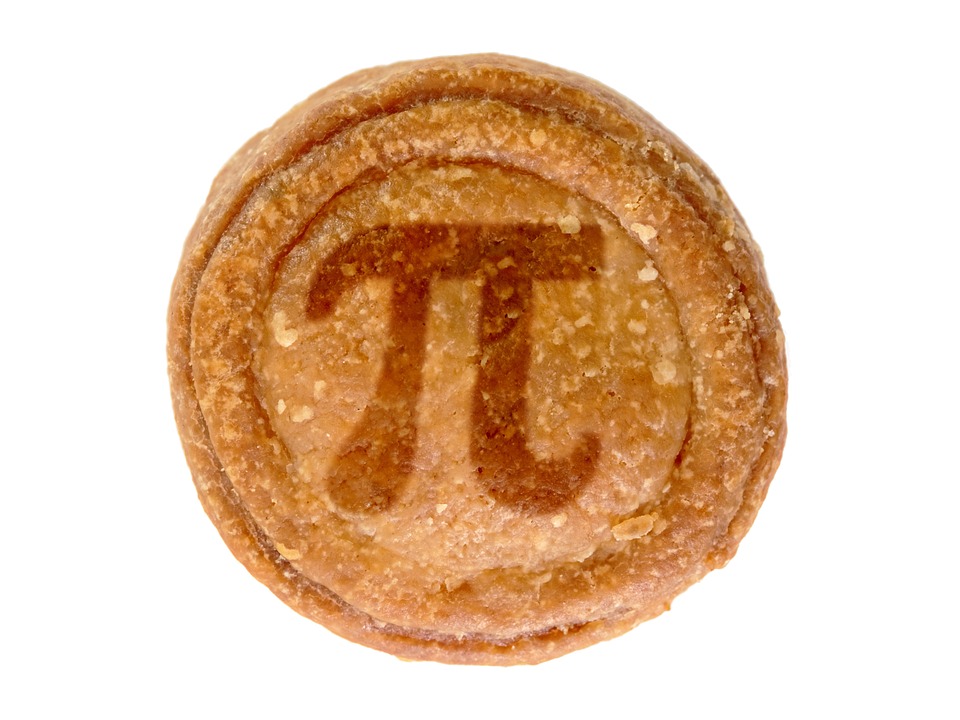
Python’s map function is a powerful tool that allows you to apply a function to each element of an iterable object, such as a list or a tuple. It provides a concise and efficient way to perform operations on multiple elements simultaneously, resulting in improved performance and code readability.
The basic syntax of the map function is as follows:
“` python
map(function, iterable)
“`
The `function` parameter represents the function that you want to apply to each element of the `iterable` object. It can be a built-in Python function or a custom-defined function. The `iterable` parameter is the object on which you want to perform the operation.
One of the key advantages of the map function is that it eliminates the need for explicit loops. Instead of writing a loop to iterate over each element and apply the function, you can simply use the map function and let it handle the iteration internally. This leads to cleaner and more concise code.
Let’s take a closer look at how the map function works under the hood. When you call the map function, it returns an iterator object that generates the results of applying the function to each element of the iterable object. It does not compute the results immediately; instead, it computes them on-the-fly as you request them.
This lazy evaluation approach has several benefits. First, it saves memory by not storing the entire result set in memory at once. Instead, it generates the results one at a time, only when needed. This is particularly useful when dealing with large datasets or when memory is limited.
Second, the lazy evaluation approach allows for efficient parallelization. When you apply the map function to a large iterable object, it can distribute the workload across multiple cores or processors, speeding up the computation. This can lead to significant performance improvements, especially when dealing with computationally intensive tasks.
To further boost performance, you can combine the map function with other built-in functions like filter and reduce. These functions allow you to filter out elements from the iterable and perform reductions, respectively. By chaining these functions together, you can create complex data processing pipelines that are both efficient and readable.
Here’s an example to illustrate the power of the map function:
“` python
numbers = [1, 2, 3, 4, 5]
# Squaring each number using map function
squared_numbers = list(map(lambda x: x**2, numbers))
print(squared_numbers)
“`
Output:
“`
[1, 4, 9, 16, 25]“`
In this example, we create a list of numbers and use the map function to square each number. The lambda function `lambda x: x**2` is applied to each element of the `numbers` list, resulting in a new list of squared numbers. By converting the map object to a list, we can easily access and manipulate the results.
In conclusion, Python’s map function is a powerful tool for boosting performance and improving code readability. It allows you to apply a function to each element of an iterable object, eliminating the need for explicit loops and enabling efficient parallelization. By understanding its inner workings and combining it with other built-in functions, you can unleash the full potential of the map function and optimize your code.