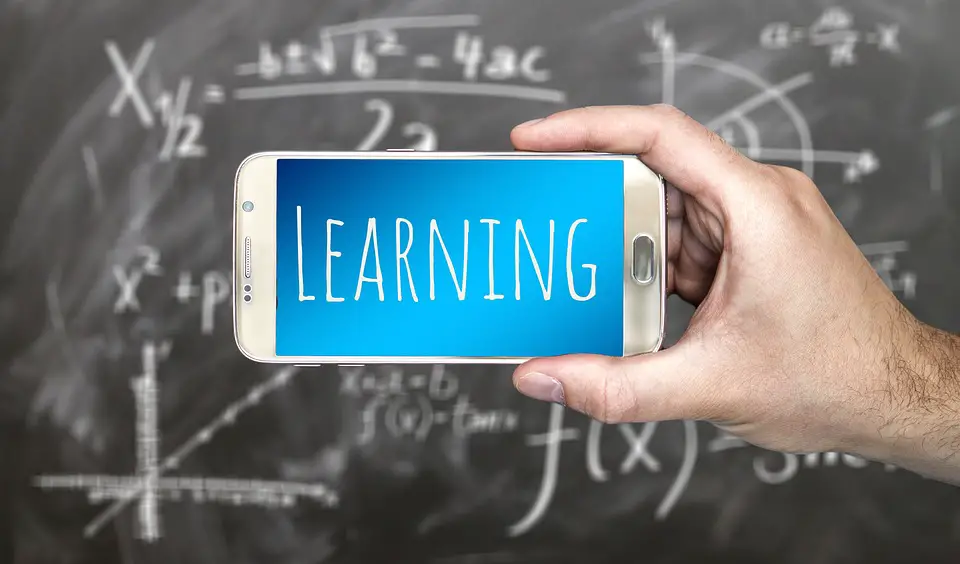
Python’s range function is a powerful tool that can help developers boost the performance of their code. By understanding how to use range effectively, developers can optimize their code for better speed and efficiency. In this article, we will discuss best practices for using range in Python to improve performance.
1. Use range instead of creating lists
One common mistake that developers make is creating a list of numbers using range instead of using the range function itself. For example, instead of using range(1000000), some developers may mistakenly write list(range(1000000)). This creates unnecessary overhead and consumes memory since it generates a list of numbers in memory. Instead, it is more efficient to use range directly without creating a list.
2. Use xrange in Python 2
In Python 2, there is a function called xrange that is similar to range but generates numbers lazily. This means that xrange does not create a list of numbers in memory but generates them on the fly when needed. In Python 3, range behaves like the old xrange function, so there is no need to use xrange in Python 3.
3. Use range with a step parameter
Range allows specifying a step parameter to generate numbers at a given interval. This can be useful for iterating over a range of numbers with a specific step size. For example, range(0, 10, 2) will generate numbers from 0 to 10 with a step size of 2 (i.e., 0, 2, 4, 6, 8).
4. Avoid unnecessary calculations inside loops
When using range in loops, it is important to avoid unnecessary calculations inside the loop body. For example, instead of calculating the length of a range inside a loop, it is better to calculate it once outside the loop and store it in a variable. This can help improve performance by reducing the number of computations inside the loop.
5. Consider using range for indexing
Range can also be used for indexing elements in a list or other data structures. By using range to generate index values, developers can access elements in a data structure more efficiently. For example, instead of using a while loop with an index variable, developers can use range to iterate over index values directly.
In conclusion, range is a powerful function in Python that can help developers boost the performance of their code. By following best practices for using range effectively, developers can optimize their code for better speed and efficiency. By avoiding unnecessary list creation, using xrange in Python 2, specifying a step parameter, avoiding unnecessary calculations inside loops, and using range for indexing, developers can take advantage of range to improve the performance of their Python code.