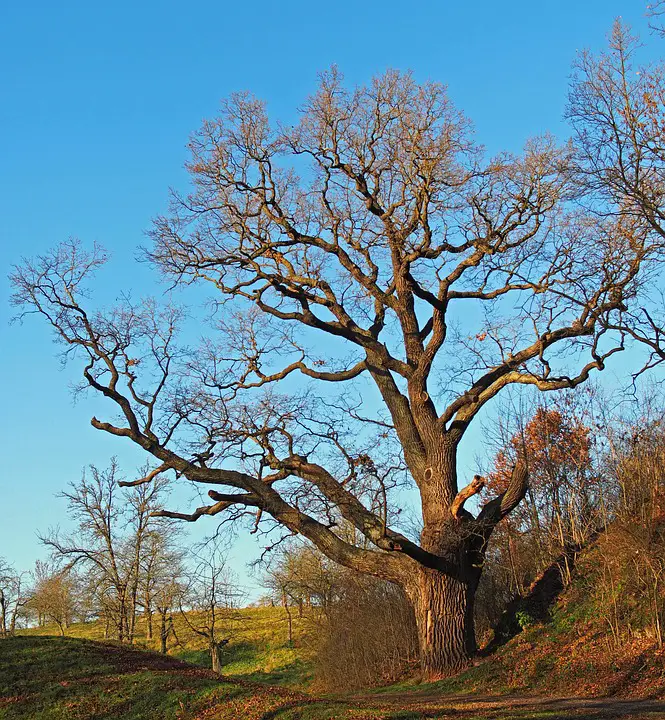
Python is a versatile and powerful programming language that is widely used across various industries and fields. One of the key features of Python is its built-in data structures, which allow for efficient and effective data manipulation. One such data structure is the set, which is a collection of unique and unordered elements.
Sets in Python are incredibly useful for tasks such as removing duplicates from a list, performing set operations (such as union, intersection, and difference), and checking for membership of an element. In this article, we will explore some essential functions and methods that will help you boost your Python skills with sets.
Creating a Set
To create a set in Python, you can use curly braces {} with comma-separated elements inside, or you can use the set() constructor with a list as an argument. For example:
“` python
my_set = {1, 2, 3, 4, 5}
print(my_set)
my_set = set([1, 2, 3, 4, 5])
print(my_set)
“`
Adding Elements to a Set
To add elements to a set, you can use the add() method. For example:
“` python
my_set = {1, 2, 3}
my_set.add(4)
print(my_set)
“`
Removing Elements from a Set
To remove elements from a set, you can use the remove() method. For example:
“` python
my_set = {1, 2, 3, 4}
my_set.remove(4)
print(my_set)
“`
Set Operations
Sets in Python support various set operations, such as union, intersection, and difference. These operations can be performed using the corresponding methods. For example:
“` python
set1 = {1, 2, 3, 4}
set2 = {3, 4, 5, 6}
# Union
union_set = set1.union(set2)
print(union_set)
# Intersection
intersection_set = set1.intersection(set2)
print(intersection_set)
# Difference
difference_set = set1.difference(set2)
print(difference_set)
“`
Checking for Membership
You can check if an element is present in a set using the in keyword. For example:
“` python
my_set = {1, 2, 3, 4}
if 3 in my_set:
print(“3 is in the set”)
“`
Iterating over a Set
You can iterate over a set using a for loop. For example:
“` python
my_set = {1, 2, 3, 4}
for element in my_set:
print(element)
“`
Conclusion
Sets are a powerful data structure in Python that can help you streamline your code and perform various operations efficiently. By mastering the essential functions and methods of sets, you can boost your Python skills and become a more proficient programmer. So, start practicing with sets and unleash their full potential in your Python projects.