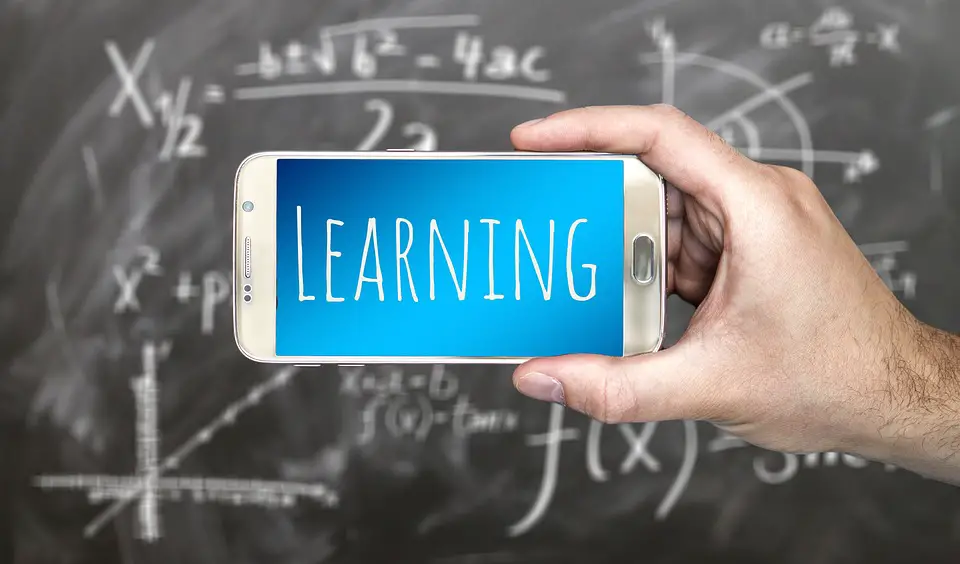
Python is a versatile and powerful programming language that is widely used in various fields such as web development, data science, and artificial intelligence. One of the most commonly used data structures in Python is the list, which is a collection of items that can be of any data type. Lists are used to store and manipulate data efficiently, and mastering the various list tricks in Python can greatly boost your coding skills.
Here are some useful Python list tricks that can help you become a more efficient and proficient programmer:
1. List Comprehension: List comprehension is a concise and elegant way to create lists in Python. It allows you to generate new lists by applying an expression to each item in an existing list. For example, you can create a list of squared numbers from 1 to 10 using list comprehension like this: squares = [x**2 for x in range(1, 11)]
2. List Slicing: List slicing is a powerful feature in Python that allows you to extract a subset of elements from a list. You can specify a start index, end index, and step size to create a new list with the desired elements. For example, you can extract every other element from a list like this: even_numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] even_numbers = even_numbers[::2]
3. List Concatenation: You can easily combine two or more lists into a single list using the concatenation operator (+). This is useful when you need to merge multiple lists together. For example, you can concatenate two lists like this: list1 = [1, 2, 3] list2 = [4, 5, 6] merged_list = list1 + list2
4. List Sorting: Python provides a built-in function called sort() that allows you to sort a list in ascending or descending order. You can also use the sorted() function to create a new sorted list without modifying the original list. For example, you can sort a list of numbers like this: numbers = [3, 1, 4, 1, 5, 9, 2, 6, 5] numbers.sort() sorted_numbers = sorted(numbers)
5. List Filtering: You can filter a list based on certain criteria using list comprehension. This allows you to create a new list that contains only the elements that meet the specified condition. For example, you can filter out even numbers from a list like this: numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] even_numbers = [x for x in numbers if x % 2 == 0]
By mastering these Python list tricks, you can become a more efficient and proficient programmer. These tricks will help you manipulate and process lists more effectively, allowing you to write cleaner and more concise code. Practice using these tricks in your projects, and you will see a significant improvement in your coding skills.