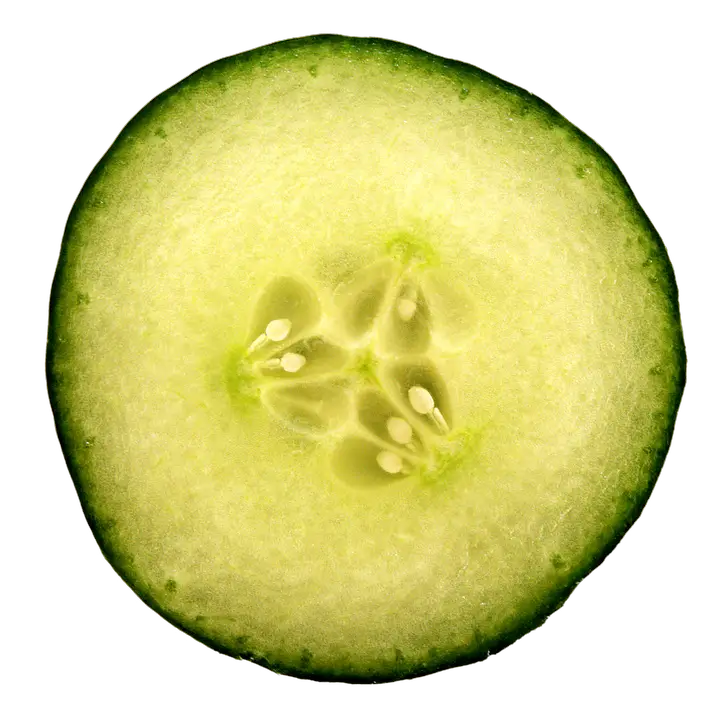
Python lists are a powerful data structure that allows you to store and manipulate collections of data in a flexible and efficient way. While the basics of working with lists in Python are relatively straightforward, there are a number of advanced techniques that can help you take your list manipulation skills to the next level. In this article, we will explore some of these advanced techniques for working with Python lists.
1. List Comprehensions
List comprehensions are a concise and powerful way to create lists in Python. They allow you to create a new list by applying an expression to each item in an existing list. For example, you can use a list comprehension to create a new list that contains the squares of all the numbers in an existing list:
“` python
numbers = [1, 2, 3, 4, 5]
squares = [x**2 for x in numbers]
print(squares) # Output: [1, 4, 9, 16, 25]
“`
List comprehensions can also be used to filter out elements from a list that don’t meet a certain condition. For example, you can use a list comprehension to create a new list that contains only the even numbers from an existing list:
“` python
numbers = [1, 2, 3, 4, 5]
evens = [x for x in numbers if x % 2 == 0]
print(evens) # Output: [2, 4]
“`
2. The zip() Function
The zip() function in Python allows you to combine multiple lists into a single list of tuples. Each tuple contains the corresponding elements from each of the input lists. For example:
“` python
names = [‘Alice’, ‘Bob’, ‘Charlie’]
ages = [25, 30, 35]
zipped = list(zip(names, ages))
print(zipped) # Output: [(‘Alice’, 25), (‘Bob’, 30), (‘Charlie’, 35)]
“`
The zip() function is particularly useful when you need to iterate over multiple lists in parallel. You can use the zip() function in conjunction with a for loop to iterate over the elements of each list simultaneously:
“` python
for name, age in zip(names, ages):
print(f'{name} is {age} years old’)
“`
3. Sorting Lists
Sorting lists is a common task in Python, and the built-in sorted() function makes it easy to sort lists in a variety of ways. By default, the sorted() function sorts lists in ascending order. For example:
“` python
numbers = [5, 2, 8, 1, 3]
sorted_numbers = sorted(numbers)
print(sorted_numbers) # Output: [1, 2, 3, 5, 8]
“`
You can also use the reverse parameter of the sorted() function to sort lists in descending order:
“` python
sorted_numbers_desc = sorted(numbers, reverse=True)
print(sorted_numbers_desc) # Output: [8, 5, 3, 2, 1]
“`
4. Removing Duplicates
If you have a list that contains duplicate elements and you want to remove them, you can use the set() function in Python. The set() function creates a new set object from the elements of the input list, which automatically removes any duplicates. You can then convert the set back to a list using the list() function. For example:
“` python
numbers = [1, 2, 3, 2, 1, 4, 5, 4]
unique_numbers = list(set(numbers))
print(unique_numbers) # Output: [1, 2, 3, 4, 5]
“`
In this article, we have explored some advanced techniques for working with Python lists, including list comprehensions, the zip() function, sorting lists, and removing duplicates. By mastering these techniques, you can become more efficient and effective at manipulating lists in Python. Experiment with these techniques in your own code to see how they can help you solve complex problems and write cleaner, more concise code.