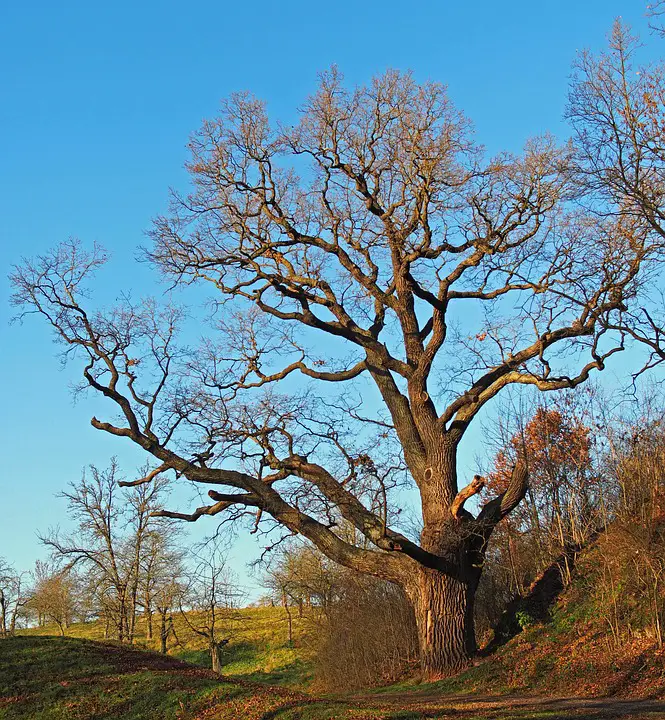
Text processing is a common task in programming, and Python is a popular choice for handling this task due to its simplicity and powerful built-in functions. One such function is the `split()` method, which is used to split a string into a list based on a specified separator. While the basic usage of `split()` is straightforward, there are advanced techniques that can be employed to handle more complex text processing tasks.
One advanced technique for using `split()` in text processing is to specify multiple separators. By default, `split()` will split a string based on whitespace characters such as spaces, tabs, and newlines. However, you can specify multiple separators by passing a list of strings to the `split()` method. For example, if you want to split a string based on both spaces and commas, you can use the following code:
“` python
text = “Hello, world! How are you today?”
words = text.split([‘ ‘, ‘,’])
print(words)
“`
This will output `[‘Hello’, ‘world!’, ‘How’, ‘are’, ‘you’, ‘today?’]`, where the string has been split based on both spaces and commas.
Another advanced technique is to use regular expressions as the separator in the `split()` method. Regular expressions are a powerful tool for pattern matching in strings, and can be used to split a string based on complex patterns. For example, if you want to split a string based on any sequence of non-alphanumeric characters, you can use the following code:
“` python
import re
text = “Hello!world123How$are*you#today?”
words = re.split(r’\W+’, text)
print(words)
“`
This will output `[‘Hello’, ‘world123How’, ‘are’, ‘you’, ‘today’]`, where the string has been split based on any sequence of non-alphanumeric characters.
Additionally, you can use the `maxsplit` parameter in the `split()` method to limit the number of splits that are performed. This can be useful when you only want to split the string a certain number of times. For example, if you want to split a string into two parts based on the first occurrence of a space character, you can use the following code:
“` python
text = “Hello world! How are you today?”
words = text.split(‘ ‘, 1)
print(words)
“`
This will output `[‘Hello’, ‘world! How are you today?’]`, where the string has been split into two parts based on the first space character.
In conclusion, the `split()` method in Python is a powerful tool for text processing, and there are several advanced techniques that can be used to handle more complex tasks. By specifying multiple separators, using regular expressions, and utilizing the `maxsplit` parameter, you can effectively split strings in a variety of ways to suit your text processing needs.