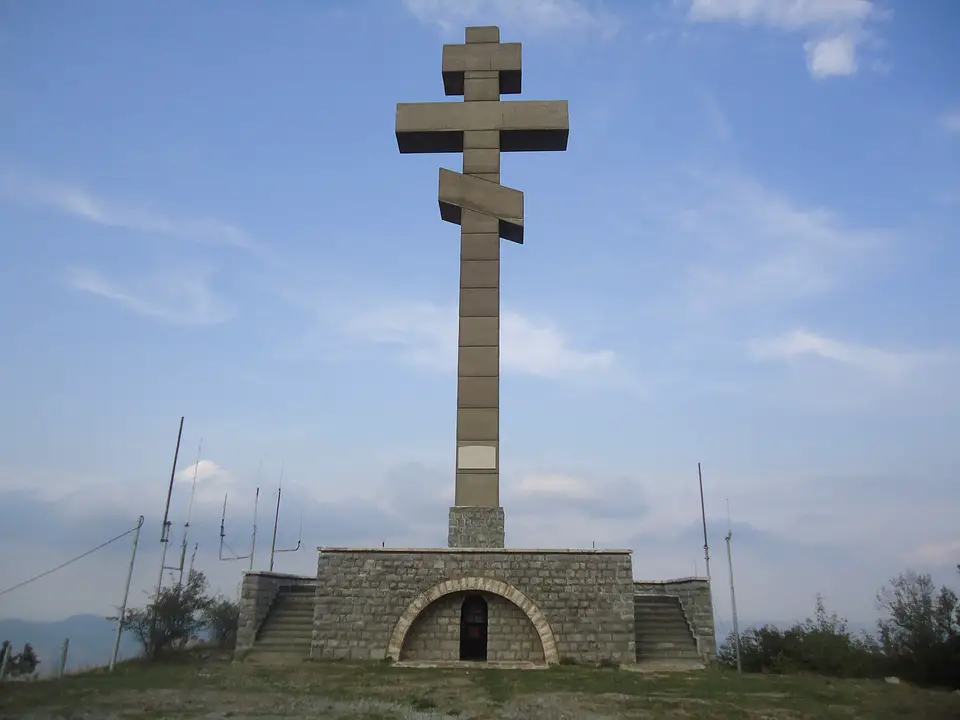
Python sets are a powerful data structure that provide a unique way to store and manipulate data. Sets are unordered collections of unique elements, meaning that each element in a set is unique and does not have any duplicate values. Working with sets in Python can be incredibly useful, but it’s important to understand how to effectively use them in order to maximize their benefits. Here are five essential tips for working with Python sets:
1. Creating a Set:
To create a set in Python, you can simply use curly braces {} with comma-separated values inside. For example, to create a set of numbers, you can write:
“` python
my_set = {1, 2, 3, 4, 5}
“`
Alternatively, you can also use the set() function to create a set from a list or tuple. For example:
“` python
my_list = [1, 2, 3, 4, 5]
my_set = set(my_list)
“`
2. Adding and Removing Elements:
You can add elements to a set using the add() method. For example:
“` python
my_set.add(6)
“`
To remove an element from a set, you can use the remove() method. For example:
“` python
my_set.remove(3)
“`
It’s important to note that if you try to remove an element that does not exist in the set, a KeyError will be raised. To avoid this, you can use the discard() method instead, which will not raise an error if the element is not present.
3. Set Operations:
Python sets support various set operations such as union, intersection, difference, and symmetric difference. These operations can be performed using built-in methods like union(), intersection(), difference(), and symmetric_difference(). For example:
“` python
set1 = {1, 2, 3, 4, 5}
set2 = {4, 5, 6, 7, 8}
# Union
print(set1.union(set2))
# Intersection
print(set1.intersection(set2))
# Difference
print(set1.difference(set2))
# Symmetric Difference
print(set1.symmetric_difference(set2))
“`
4. Set Comprehensions:
Just like lists and dictionaries, sets also support comprehensions in Python. Set comprehensions allow you to create sets using a more concise syntax. For example, you can create a set of squares of numbers from 1 to 5 using set comprehension:
“` python
my_set = {x**2 for x in range(1, 6)}
“`
5. Checking Membership:
You can check if an element is present in a set using the in keyword. For example:
“` python
my_set = {1, 2, 3, 4, 5}
if 3 in my_set:
print(“3 is in the set”)
“`
Alternatively, you can also use the issubset() and issuperset() methods to check if a set is a subset or superset of another set. For example:
“` python
set1 = {1, 2, 3}
set2 = {1, 2, 3, 4, 5}
print(set1.issubset(set2))
print(set2.issuperset(set1))
“`
In conclusion, Python sets are a versatile data structure that can be incredibly useful in various programming scenarios. By following these essential tips for working with Python sets, you can effectively manipulate and utilize sets in your Python programs.