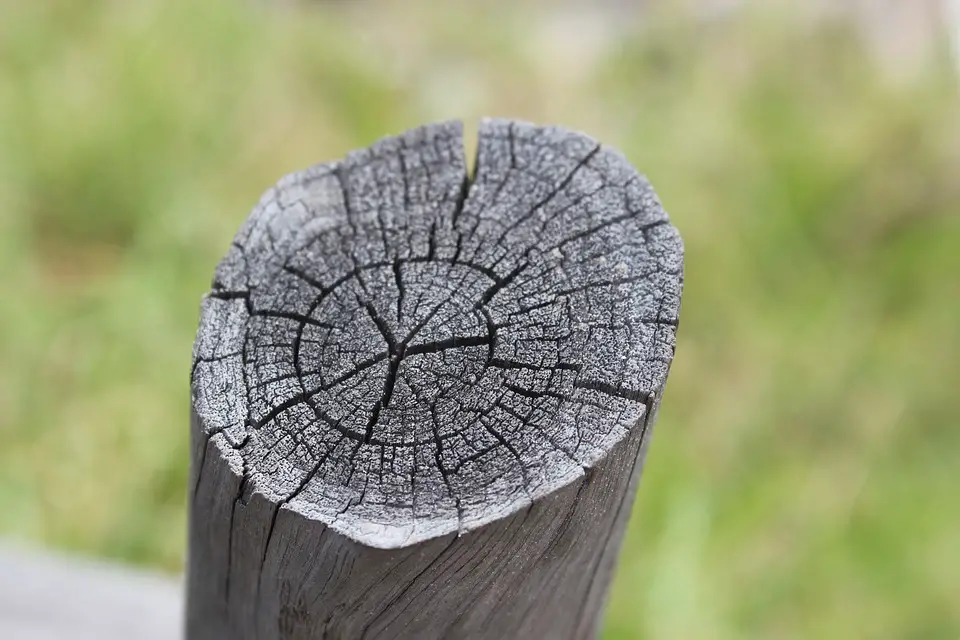
Python’s range function is a powerful tool that allows programmers to generate a sequence of numbers quickly and efficiently. While it is commonly used for creating loops in code, there are many creative ways to utilize the range function in your projects. In this article, we will explore five innovative ways to use Python’s range function in your code.
1. Creating custom sequences:
One creative way to use Python’s range function is to create custom sequences of numbers. By specifying the start, stop, and step parameters, you can generate a sequence that meets your specific requirements. For example, you can create a sequence of even numbers by setting the step parameter to 2, or you can generate a sequence of multiples of 5 by setting the step parameter to 5.
“` python
for i in range(0, 10, 2):
print(i)
“`
2. Iterating over a sequence in reverse:
Another useful way to use the range function is to iterate over a sequence of numbers in reverse. By setting the step parameter to -1, you can loop through a range of numbers in reverse order. This can be helpful when you need to process a list or array in reverse.
“` python
for i in range(10, 0, -1):
print(i)
“`
3. Generating indices for a list:
When working with lists or arrays in Python, you may need to access elements by their index. The range function can be used to generate a sequence of indices that correspond to the elements in a list. This can simplify your code and make it more readable.
“` python
my_list = [‘a’, ‘b’, ‘c’, ‘d’, ‘e’]
for i in range(len(my_list)):
print(my_list[i])
“`
4. Creating nested loops:
The range function can also be used to create nested loops in Python. By nesting multiple range functions within each other, you can generate a two-dimensional grid of numbers that can be used for processing data or performing calculations.
“` python
for i in range(3):
for j in range(3):
print(f'({i}, {j})’)
“`
5. Generating arithmetic sequences:
Lastly, the range function can be used to generate arithmetic sequences of numbers. By setting the start, stop, and step parameters appropriately, you can create a sequence of numbers that follow a specific arithmetic pattern. This can be useful for generating test data or simulating mathematical functions.
“` python
for i in range(0, 10, 2):
print(i)
“`
In conclusion, Python’s range function is a versatile tool that can be used in a variety of creative ways in your code. By exploring the different ways to utilize the range function, you can improve the efficiency and readability of your Python programs. Experiment with these techniques in your own projects to discover new ways to leverage the power of the range function.