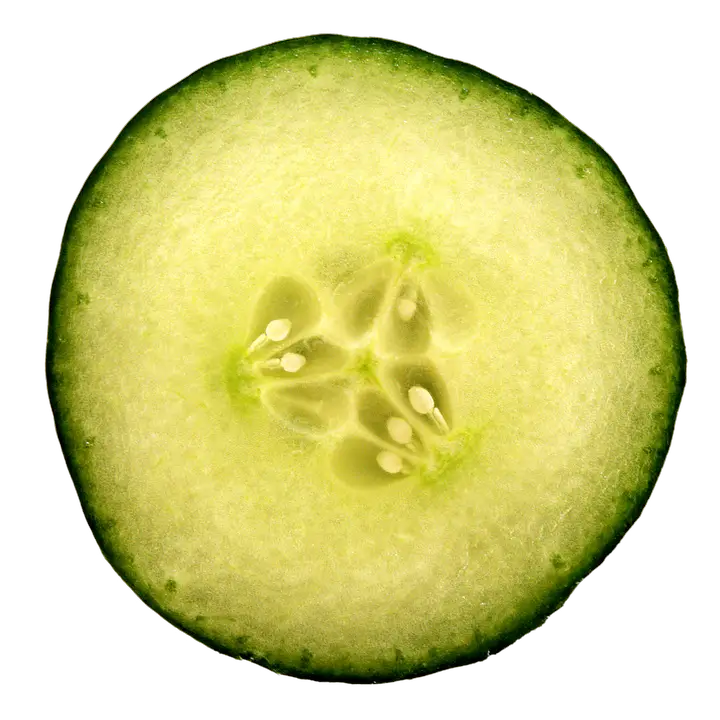
Python lists are a versatile and powerful data structure that can be used for a wide range of tasks in programming. Whether you’re a beginner or an experienced programmer, working with lists in Python can sometimes be challenging. To help you make the most out of Python lists, here are 10 tips and tricks to keep in mind:
1. Use list comprehension: List comprehension is a concise way to create lists in Python. It allows you to generate a new list by applying an expression to each element of an existing list. For example, you can create a new list of squared numbers from an existing list using list comprehension like this: `squared_numbers = [x**2 for x in numbers]`.
2. Avoid using loops to modify lists: Modifying lists in Python using loops can be inefficient and error-prone. Instead, use built-in functions like `map()` or `filter()` to transform lists in a more concise and efficient manner.
3. Use slicing to manipulate lists: Slicing is a powerful feature in Python that allows you to extract specific elements from a list. For example, you can extract a sublist from a list using slicing like this: `sublist = my_list[start_index:end_index]`.
4. Use the `append()` method to add elements to a list: The `append()` method is a convenient way to add elements to the end of a list. For example, you can add a new element to a list like this: `my_list.append(new_element)`.
5. Use the `extend()` method to add multiple elements to a list: If you want to add multiple elements to a list, you can use the `extend()` method. This method takes an iterable as an argument and adds each element to the end of the list. For example, you can add multiple elements to a list like this: `my_list.extend([element1, element2, element3])`.
6. Use the `index()` method to find the index of an element in a list: If you want to find the index of a specific element in a list, you can use the `index()` method. This method returns the index of the first occurrence of the specified element in the list. For example, you can find the index of an element in a list like this: `index = my_list.index(element)`.
7. Use the `remove()` method to delete an element from a list: If you want to remove a specific element from a list, you can use the `remove()` method. This method removes the first occurrence of the specified element from the list. For example, you can remove an element from a list like this: `my_list.remove(element)`.
8. Use the `pop()` method to remove and return an element from a list: If you want to remove and return an element from a list, you can use the `pop()` method. This method removes the element at the specified index from the list and returns it. For example, you can remove and return an element from a list like this: `element = my_list.pop(index)`.
9. Use the `sort()` method to sort a list in place: If you want to sort a list in place, you can use the `sort()` method. This method sorts the elements of the list in ascending order. For example, you can sort a list like this: `my_list.sort()`.
10. Use the `reverse()` method to reverse the order of elements in a list: If you want to reverse the order of elements in a list, you can use the `reverse()` method. This method reverses the order of elements in the list. For example, you can reverse the order of elements in a list like this: `my_list.reverse()`.
By following these tips and tricks, you can effectively work with Python lists and make your code more efficient and readable. Lists are a fundamental data structure in Python, and mastering them will greatly enhance your programming skills. Happy coding!