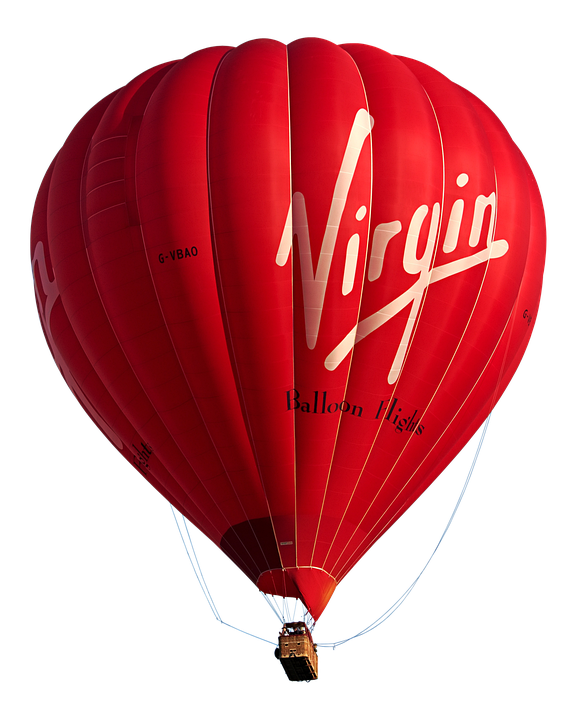
The Go programming language, also known as Golang, has been gaining popularity among developers for its simplicity, efficiency, and performance. If you’re new to Go and looking to sharpen your skills, here are 10 must-try Go programming language examples for beginners.
1. Hello World
The classic “Hello World” program is a great way to get started with any programming language. In Go, it’s as simple as:
“`go
package main
import “fmt”
func main() {
fmt.Println(“Hello, World!”)
}
“`
2. Variables and Constants
Understanding how to declare and use variables and constants is essential in any programming language. Here’s an example in Go:
“`go
package main
import “fmt”
func main() {
var x int = 5
const y = 10
fmt.Println(x)
fmt.Println(y)
}
“`
3. Functions
Functions are the building blocks of any program. Here’s a basic function example in Go:
“`go
package main
import “fmt”
func add(x int, y int) int {
return x + y
}
func main() {
fmt.Println(add(3, 5))
}
“`
4. Arrays and Slices
Arrays and slices are essential data structures in Go. Here’s an example of how to use them:
“`go
package main
import “fmt”
func main() {
numbers := [5]int{1, 2, 3, 4, 5}
fmt.Println(numbers)
slice := numbers[1:4]
fmt.Println(slice)
}
“`
5. Loops
Loops are used to iterate through data structures or execute a block of code repeatedly. Here’s an example of a for loop in Go:
“`go
package main
import “fmt”
func main() {
for i := 0; i 5 {
fmt.Println(“x is greater than 5”)
} else {
fmt.Println(“x is less than or equal to 5”)
}
}
“`
7. Structs
Structs are used to group related data together. Here’s an example of how to define and use a struct in Go:
“`go
package main
import “fmt”
type Person struct {
Name string
Age int
}
func main() {
p := Person{Name: “Alice”, Age: 30}
fmt.Println(p)
}
“`
8. Pointers
Pointers are variables that store the memory address of another variable. Here’s an example of how to use pointers in Go:
“`go
package main
import “fmt”
func main() {
x := 10
p := &x
fmt.Println(*p)
}
“`
9. Error Handling
Error handling is important in any program to handle unexpected situations. Here’s an example of how to handle errors in Go:
“`go
package main
import (
“fmt”
“errors”
)
func divide(x, y float64) (float64, error) {
if y == 0 {
return 0, errors.New(“cannot divide by zero”)
}
return x / y, nil
}
func main() {
result, err := divide(10, 0)
if err != nil {
fmt.Println(“Error:”, err)
} else {
fmt.Println(“Result:”, result)
}
}
“`
10. Goroutines
Goroutines are lightweight threads that allow concurrent execution in Go. Here’s an example of how to use goroutines:
“`go
package main
import (
“fmt”
“time”
)
func sayHello() {
fmt.Println(“Hello, Go!”)
}
func main() {
go sayHello()
time.Sleep(1 * time.Second)
}
“`
These 10 examples cover some of the fundamental concepts of the Go programming language. By trying out these examples, beginners can start to build a solid foundation in Go and become more comfortable with the language. Happy coding!