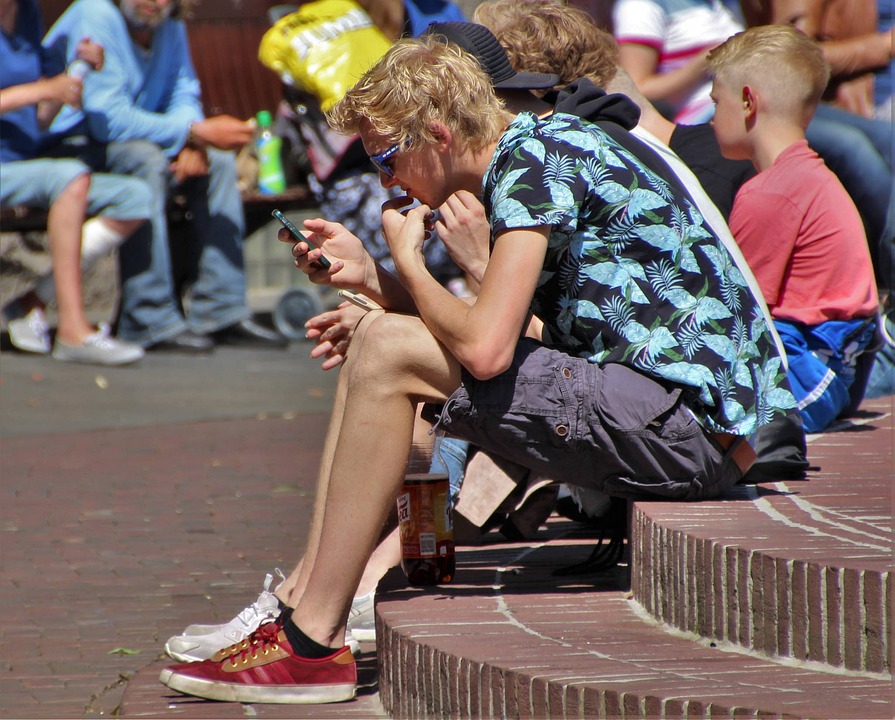
Go, also known as Golang, is a programming language developed by Google that has gained popularity in recent years for its simplicity, efficiency, and concurrency support. If you’re a developer looking to learn more about Go and its capabilities, here are 10 must-see examples that showcase the power and versatility of this language.
1. Hello, World!
The classic “Hello, World!” example is a great way to get started with any programming language, and Go is no exception. In just a few lines of code, you can create a simple program that prints out this famous phrase to the console.
“`go
package main
import “fmt”
func main() {
fmt.Println(“Hello, World!”)
}
“`
2. Variables and Constants
In Go, you can declare variables using the `var` keyword and constants using the `const` keyword. Here’s an example that demonstrates how to declare and initialize variables and constants:
“`go
package main
import “fmt”
func main() {
var name string = “John”
const age = 30
fmt.Printf(“Name: %s, Age: %d\n”, name, age)
}
“`
3. Arrays and Slices
Arrays and slices are fundamental data types in Go that allow you to store collections of elements. Here’s an example that shows how to declare and manipulate arrays and slices:
“`go
package main
import “fmt”
func main() {
var numbers [5]int
numbers[0] = 1
numbers[1] = 2
numbers[2] = 3
fmt.Println(“Array:”, numbers)
var colors = []string{“red”, “green”, “blue”}
colors = append(colors, “yellow”)
fmt.Println(“Slice:”, colors)
}
“`
4. Loops
Go supports various types of loops, including `for`, `while`, and `range` loops. Here’s an example that demonstrates how to use these loops to iterate over arrays and slices:
“`go
package main
import “fmt”
func main() {
numbers := []int{1, 2, 3, 4, 5}
for i := 0; i