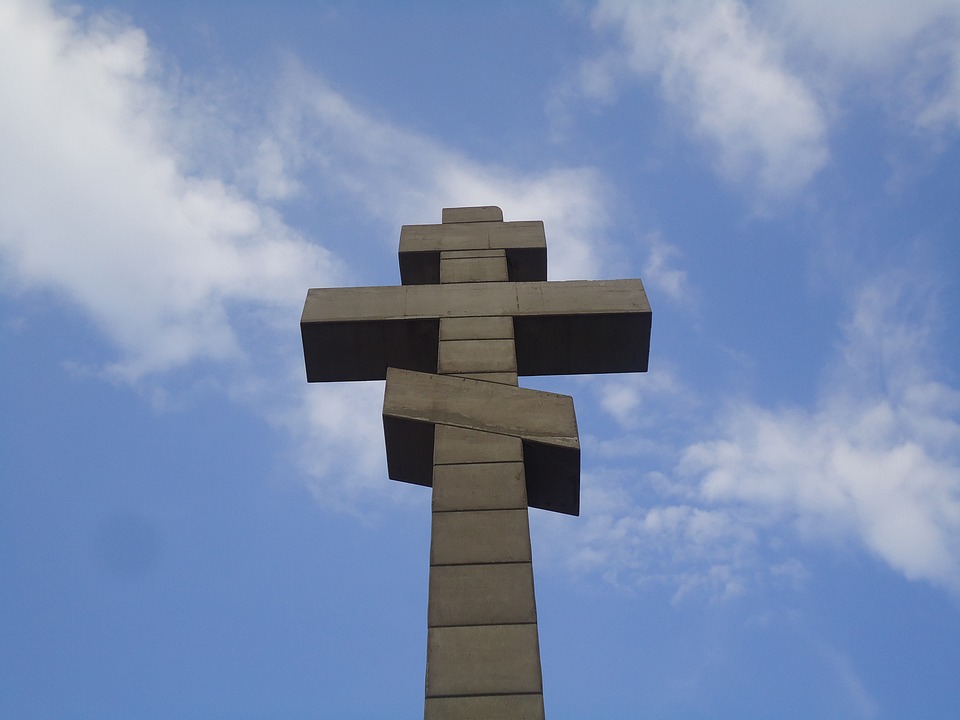
Python lists are incredibly versatile and powerful data structures that allow you to store and manipulate collections of items. Whether you are a beginner or an experienced Python programmer, there are always new tricks and techniques to learn when it comes to working with lists. In this article, we will explore 10 essential tips and tricks for manipulating Python lists, which will help you become more efficient and effective in your coding.
1. Indexing and Slicing:
One of the most basic yet powerful features of Python lists is the ability to access individual elements using their index. The first element has an index of 0, the second has an index of 1, and so on. You can also use slicing to extract a portion of a list. For example, `my_list[2:5]` will return a new list containing elements from index 2 to 4.
2. List Comprehensions:
List comprehensions are a concise and elegant way to create new lists from existing ones. They allow you to apply an expression to each element of a list and generate a new list based on the results. For example, you can use `[x**2 for x in my_list]` to create a new list where each element is the square of the corresponding element in `my_list`.
3. Adding and Removing Elements:
To add an element to the end of a list, you can use the `append()` method. If you want to insert an element at a specific index, you can use the `insert()` method. To remove an element, you can use the `remove()` method if you know the value, or the `del` statement if you know the index. For example, `my_list.remove(3)` will remove the first occurrence of the value 3 from `my_list`.
4. Sorting Lists:
Python provides the `sort()` method to sort a list in ascending order. If you want to sort the list in descending order, you can pass the `reverse=True` argument to the `sort()` method. Additionally, you can use the `sorted()` function to create a new sorted list without modifying the original list.
5. Reversing Lists:
To reverse the order of elements in a list, you can use the `reverse()` method. This will modify the original list. If you want to create a new reversed list without modifying the original list, you can use the slicing technique `my_list[::-1]`.
6. Checking for Membership:
You can easily check if an element is present in a list using the `in` operator. For example, `5 in my_list` will return `True` if 5 is in `my_list`, and `False` otherwise. This can be useful when you want to perform different actions based on whether an element is present in a list or not.
7. Counting Elements:
Python provides the `count()` method to count the number of occurrences of a specific element in a list. For example, `my_list.count(2)` will return the number of times the value 2 appears in `my_list`. This can be handy when you need to analyze the frequency of certain elements in a list.
8. Concatenating Lists:
To combine two lists into a single list, you can use the `+` operator. For example, `my_list + other_list` will return a new list that contains all the elements from `my_list` followed by all the elements from `other_list`. This operation does not modify the original lists.
9. Checking List Equality:
To check if two lists have the same elements in the same order, you can use the `==` operator. For example, `[1, 2, 3] == [1, 2, 3]` will return `True`. However, keep in mind that this only checks for equality at the top level and does not perform deep comparison.
10. Copying Lists:
When you assign a list to a new variable, both variables will refer to the same list object. If you want to create a copy of a list, you can use the `copy()` method or the slicing technique `my_list[:]`. This is useful when you want to modify one copy of the list without affecting the other.
These are just a few of the many tips and tricks for manipulating Python lists. By mastering these techniques, you will be able to handle lists more efficiently and leverage their full potential in your Python programs. So go ahead, experiment with these tips, and unlock new possibilities with Python lists.