Using Pandas to Read JSON from URL
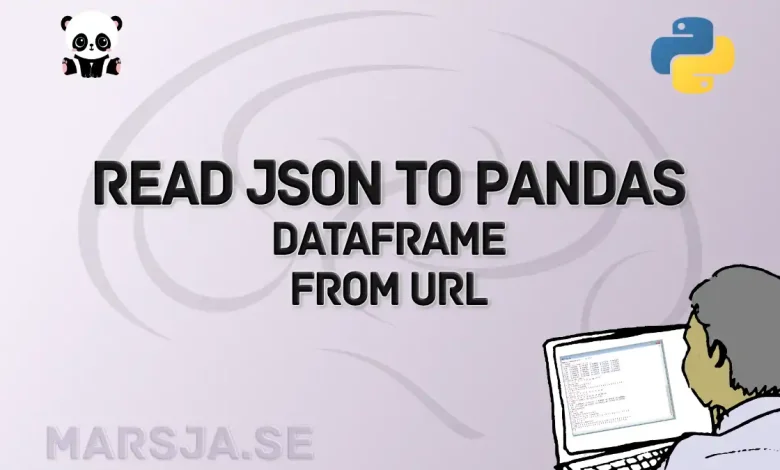
When working with data in Python, using Pandas to read JSON from URL is an excellent tool that lets you directly load JSON data from a web source into a Pandas dataframe. This tutorial will teach you the steps to accomplish this task, building upon our previous discussions on reading JSON with Python more generally.
Table of Contents
How to use Pandas to Read JSON from URL
First, let us look at a simple example of using Pandas to read JSON from a URL.
import pandas as pd
url = "http://api.open-notify.org/astros.json"
df = pd.read_json(url)
print(df)
Code language: PHP (php)
In the code chunk above, we start by importing the Pandas library. The URL variable contains the web address where the JSON data is hosted. The pd.read_json(url)
function is then used to read the JSON data from the URL and load it into a Pandas DataFrame, which is a two-dimensional labeled data structure with columns of potentially different types. Finally, print(df)
displays the DataFrame, allowing us to see the imported data in tabular format.
Now that we have seen a basic example, let us learn more about the parameters of the pd.read_json()
method to understand how we can customize the reading process.
Parameters of the read_json Method
The pd.read_json()
method has several parameters that allow you to fine-tune how the JSON data is read and converted into a dataframe. Here is an overview of the most important parameters:
path_or_buf
: The string containing the URL or the path to the JSON file. This is the source of the JSON data that will be read.orient
: Defines the expected JSON string format. Default is ‘columns’. This parameter specifies the orientation of the JSON data. Other options include ‘split’, ‘records’, ‘index’, and ‘values’.typ
: Specifies the type of object to be returned. Default is ‘frame’. This parameter can be set to ‘series’ if you want to return a Series instead of a DataFrame.dtype
: Determines whether to infer types of objects. Default is ‘None’. This parameter can be used to specify the data type for each column.convert_axes
: Whether to convert the axes to another type. Default is ‘True’. This parameter allows you to convert the axes to a specified data type.convert_dates
: List of columns to convert to dates. Default is ‘True’. This parameter can be used to specify which columns should be parsed as dates.keep_default_dates
: Whether to include default date parsers. Default is ‘True’. This parameter determines whether to use the default date parsers provided by Pandas.precise_float
: Whether to use a high precision floating point converter. Default is ‘False’. This parameter can be set to ‘True’ if you need high precision for float values.date_unit
: Unit for encoding datetime. Default is ‘None’. This parameter can be used to specify the time unit for encoding datetime objects.encoding
: Specifies the encoding to be used. Default is ‘utf-8’. This parameter determines the encoding for reading the JSON data.lines
: Whether to read the JSON file as a JSON object per line. Default is ‘False’. This parameter can be set to ‘True’ if the JSON data is in a line-delimited format.
With these parameters allows you to better control how JSON data is read and processed, enabling you to tailor the DataFrame to your needs.
Summary
To summarize, we have learned how to use Pandas to read JSON data from a URL. We explored a practical example and detailed the parameters of the pd.read_json()
method, enhancing our ability to customize the data reading process. Handling nested JSON data can be more challenging, but that will be covered in a future post.
I would appreciate it if you could share this post and leave your comments below. Your feedback is invaluable!
Resources
Here are some other reading data-related tutorials: